Dharkael Enchanter
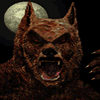
Joined: 05 Mar 2003 Posts: 593 Location: Canada
|
Posted: Mon Apr 12, 2004 12:04 am
Colour Of Word on a line |
this script was inspired by a question on the general topic
http://zuggsoft.com/forum/topic.asp?TOPIC_ID=15689
But since it is a finished script I thought I would post it here.
This script is done in JScript as Windows Script Component.
it has 8 methods or properties.
IsValidRegExp() takes a string argument of a possible valid JScript Regular Expression and returns true if it is valid and false if it isnt.
Colours is a readonly property that returns a list of the names of the colours you've set seperated by a comma.
AddColour takes two parameters the first is JScript Regular Expression string that will match an ansi colour code.
the second argument is the name or description of that code
that will be returned when you use the ColourOf or ColourAt methods.
it returns true if the colour was added and false if there was a problem creating the Regular Expression.
RemoveColour method takes one parameter a string that represents
the pattern of a previously added colour. returns true if it deleted
a colour and false if it doesn't.
RemoveColourByName takes one parameter a string that matches the name of a previously added colour. returns true if it deletes something false if it doesn't.
ClearColours method deletes all previously added colours.
ColourOf method takes two parameters the first is the ansi coloured string we want to search and the second is substring we'd like to find after the ansi information is stripped.
returns a string matching the name of the colour at the start of that substring. you should change it to return a default value
if it cant find that colour or find the substring at all.
ColourAt is like ColourOf takes two parameters first is the ansi coloured string to search and second is the zero based index
of the character that we wish to know the colour of.
the index assumes is the index of the string if no ansi information
was present.
Info on how to JScript Regular Expression Syntax can be found
here .
http://msdn.microsoft.com/library/default.asp?url=/library/en-us/script56/html/js56jsgrpregexpsyntax.asp
Sample Usage:
#TRIGGER {%e[35m(*) tells you %e[0m'(*)'} {#var WordColour %comcreate("WordColour.WSC");#if (%stripansi( "%2")) {Say @WordColour.ColourOf("%0",stripansi(%1))};Say the 6th character was @Word.ColourAt("%0",7)} "" {color}
Thats it thats all here's the code.
<?xml version="1.0" ?>
<package>
<component id="WordColour">
<?component error="true" debug="true" ?>
<registration progid="WordColour.WSC" classid="{B750A0BF-443E-4EA3-9E89-DB2B18481E3F}" description="WordColour" version="1.0">
<script language="VBScript">
<![CDATA[
Function Register()
Dim TypeLib
Set TypeLib = CreateObject("Scriptlet.TypeLib")
TypeLib.AddURL "WordColour.WSC"
TypeLib.Path = "WordColourWSC.tlb"
TypeLib.Doc = "WordColour"
TypeLib.Name = "WordColourWSC.tlb"
TypeLib.MajorVersion = 1
TypeLib.MinorVersion = 0
TypeLib.Write
End Function
Function Unregister()
End Function
]]>
</script>
</registration>
<public>
<property name="Colours">
<get/>
</property>
<method name="AddColour">
</method>
<method name="RemoveColour">
<parameter name="theclr"/>
</method>
<method name="ColourOf">
<parameter name="ansiStr"/>
<parameter name="trgStr"/>
</method>
<method name="RemoveColourByName">
<parameter name="name"/>
</method>
<method name="ClearColours">
</method>
<method name="IsValidRegExp">
<parameter name="regPtrn"/>
</method>
<method name="ColourAt">
<parameter name="ansiStr"/>
<parameter name="iPos"/>
</method>
</public>
<script id="WordColour" language="JScript">
<![CDATA[
var description = new WordColour;
var sre = new RegExp();
sre.compile(/x1Bx5B(?:(?:0|1);)?(?:0|(?:3[0-7]))m/);
//used for the stripansi function
function WordColour()
{
this.ColourAt = ColourAt;
this.IsValidRegExp = IsValidRegExp;
this.ClearColours = ClearColours;
this.RemoveColourByName = RemoveColourByName;
this.ColourOf = ColourOf;
this.RemoveColour = RemoveColour;
this.AddColour = AddColour;
this.get_Colours = get_Colours;
}
var Colours = new Array();
function get_Colours()
{
var tmp =new Array();
for(lx=0;lx<Colours.length;lx++)
tmp.push(Colours[lx].First);
return tmp.join();
}
function AddColour(clrPtrn,name)
{
try{
var tmp = new RegExp();
tmp.compile(clrPtrn);
Colours.push(new Pair(name,tmp));
}catch(e){return false;}
return true;
}
function RemoveColour(clrPtrn)
{
for (lx=0;lx<Colours.length;lx++)
{
if(Colours[lx].Second.source==tmp)
{
delete Colours[lx];
return true;
}
}
return false;
}
function RemoveColourByName(name)
{
for (lx=0;lx<Colours.length;lx++)
{
if(Colours[lx].First==name)
{
delete Colours[lx];
return true;
}
}
return false;
}
function ColourOf(ansiStr, trgStr)
{
trgStr= stripAnsi(trgStr); //just incase we passed in some unwanted ansi values with this string
var ind = stripAnsi(ansiStr).indexOf(trgStr);
if(ind<0) // that substring isnt in the ansiStr
return "No Matching Substring"; // should either throw an Error return null or use a default value
var eclrs = EnumColours(ansiStr);
while((c = eclrs.pop())!=null)
{
if(c.First<=ind)
{
for(i=0;i<Colours.length;i++)
{
if(Colours[i].Second.test(c.Second))
return Colours[i].First;
}
}
}
return "Cant Find That Colour"; // String was there but The previous colour wasnt in our list
} // Could return a default value or null
function EnumColours(txt)
{
var re = new RegExp();
re.compile(/x1Bx5B(?:(?:0|1);)?(?:0|(?:3[0-7]))m/g); //we set the global flag (the terminal 'g')
var csum =0;
var myar = new Array();
while(true)
{
var ar = re.exec(txt);
if(ar==null)
break;
var pStr = txt.substring(ar.index,ar.lastIndex)
myar.push(new Pair(ar.index-csum,pStr));
csum += pStr.length;
}
return myar;
}
function Pair(First,Second)
{
this.First = First;
this.Second = Second;
}
function stripAnsi(str)
{
return (new String(str)).split(sre).join("");
}
//Default Colours should change to match your needs
AddColour("\x1B\x5B0m","Clear");
AddColour("\x1B\x5B(?:0;)?(?:34m)","Blue");
AddColour("\x1B\x5B1;34m","Bright Blue");
AddColour("\x1B\x5B(?:0;)?(?:36m)","Cyan");
AddColour("\x1B\x5B(?:1;36m)","Bright Cyan");
AddColour("\x1B\x5B(?:0;)?(?:32m)","Green");
AddColour("\x1B\x5B(?:1;32m)","Bright Green");
AddColour("\x1B\x5B(?:0;)?(?:35m)","Magenta");
AddColour("\x1B\x5B(?:1;35m)","Bright Magenta");
AddColour("\x1B\x5B(?:0;)?(?:31m)","Red");
AddColour("\x1B\x5B(?:1;31m)","Bright Red");
AddColour("\x1B\x5B(?:0;)?(?:33m)","Yellow");
AddColour("\x1B\x5B(?:1;33m)","Bright Yellow");
AddColour("\x1B\x5B(?:1;30m)","Grey");
function ClearColours()
{
Colours = new Array();
}
function IsValidRegExp(regPtrn)
{
try{
new RegExp(regPtrn);
}
catch(e){return false;}
return true;
}
function ColourAt(ansiStr, iPos)
{
iPos = parseInt(iPos);
if(isNaN(iPos)) //its not a number so we'll assign it a default val of 0
iPos =0;
if(iPos<0)
iPos=0; //cant look before the string can we
i = stripAnsi(ansiStr).length;
if(iPos>=i) // nor after it
iPos = (i-1);
var eclrs = EnumColours(ansiStr);
while((c = eclrs.pop())!=null)
{
if(c.First<=iPos)
{
for(i=0;i<Colours.length;i++)
{
if(Colours[i].Second.test(c.Second))
return Colours[i].First;
}
}
}
return "This string has no Colour";//as usual could return some default value
}
]]>
</script>
</component>
</package>
//Watch out for word the word wrap. |
|