 |
eupher Apprentice
Joined: 18 Jan 2001 Posts: 116 Location: USA
|
Posted: Sun Nov 17, 2013 4:43 pm
Mapper: Find vnum for room in given direction |
Is there a way to retrieve the vnum of the room in a given direction in the mapper?
For example, my current location has an east exit... how to I pull the vnum of the destination to the east?
Thanks |
|
|
 |
MattLofton GURU
Joined: 23 Dec 2000 Posts: 4834 Location: USA
|
Posted: Sun Nov 17, 2013 6:34 pm |
You can't do it directly via the various %map or %room functions, you have to do it via SQL. This is because room objects and exit objects are kept separate in the db.
1)create your db connection object
//you can see the list of db connections by using the #SQLDB command
//the connection, in this example, would appear as "mapdb -> C:\blah\blah\mapdb.dbm [sqlite-3] connected"
$mapdb = %sqldb("mapdb","c:\blah\blah\mapdb.dbm")
2)fashion your query
//this one will return all the rooms your current location exits to
$query = %sql("SELECT * FROM ExitTbl WHERE FromId="%roomkey()" AND FromId<>ToId")
//this one will only return the room your current location exits to in the specified direction
//for now, we'll assume that $direction = 3 (the dirtype that equates to east)
$query = %sql("SELECT * FROM ExitTbl WHERE FromId="%roomkey()" AND FromId<>ToId AND DirType="$direction)
In SQL, you'll have four basic operations--SELECT, INSERT, UPDATE, and DELETE. SELECT returns a list of record objects, all the others do not. SELECT has to be used via %sql(), the others have to be used via the Execute() method of your connection object. I'll leave you to hunt down the syntax for those three (w3schools.com is pretty awesome as a tutorial.)
#call $mapdb.execute("INSERT blah blah blah") |
|
_________________ EDIT: I didn't like my old signature |
|
|
 |
Daern Sorcerer
Joined: 15 Apr 2011 Posts: 809
|
Posted: Sun Nov 17, 2013 6:50 pm |
Or just use %roomlink(, e).
|
|
|
 |
eupher Apprentice
Joined: 18 Jan 2001 Posts: 116 Location: USA
|
Posted: Sun Nov 17, 2013 6:55 pm |
Thanks so much for the help. I'm still trying to sort out how to use the first approach, but the second approach leads to another question I have already...
%roomlink() returns a room number, not vnum (which I have associated with the real room numbers on the mud I play). How do you convert a mapper room number to the vnum?
I have tried:
#SHOW %roomvnum(%roomlink(,e))
and it returns a number... just not the right number for the vnum of the room to the east.
#SHOW %(roomlink(,e))
does show the right room number for the room to the east. How do I convert this to vnum correctly?
Thanks again |
|
|
 |
eupher Apprentice
Joined: 18 Jan 2001 Posts: 116 Location: USA
|
Posted: Sun Nov 17, 2013 9:23 pm |
With assistance from MattLofton's hints getting me in the right direction, I have come up with a solution that seems to solve my problem. I am quite sure that it's not the prettiest way of doing it, but it seems to work.
I created a function @getVNumFromObjId($objID) that will convert a mapper object ID (ObjectTbl.ObjId) to a vnum (ObjectTbl.RefNum):
Code: |
#SQLDB TheForestsEdge.dbm
$query = %sql(TheForestsEdge,%concat("SELECT * FROM ObjectTbl where ObjId=",$ObjId))
#IF (! $query.RecordCount() = 0) {
#RETURN $query.Item("RefNum")
} {
#RETURN -1
} |
I then created a function @getExitVNum($direction) that will return a vnum for the room in the specified direction:
Code: |
#SQLDB TheForestsEdge.dbm
#SWITCH ($direction)
(n) {$direction=0}
(e) {$direction=2}
(s) {$direction=4}
(w) {$direction=6}
(u) {$direction=8}
(w) {$direction=9}
$query = %sql(TheForestsEdge,%concat("SELECT * FROM ExitTbl WHERE FromId=",%roomkey()," AND FromId<>ToId AND DirType=",$direction))
#IF (! $query.RecordCount() = 0) {
#RETURN @getVNumFromObjId($query.Item("ToID"))
} {
#RETURN -1
} |
I hope this helps anyone who may have the same question in the future.
Thanks again for the help. |
|
|
 |
Daern Sorcerer
Joined: 15 Apr 2011 Posts: 809
|
Posted: Mon Nov 18, 2013 12:37 am |
This works for me:
Code: |
#show %roomnum(%roomlink(, e)) |
|
|
|
 |
eupher Apprentice
Joined: 18 Jan 2001 Posts: 116 Location: USA
|
Posted: Mon Nov 18, 2013 12:56 am |
Daern wrote: |
This works for me:
Code: |
#show %roomnum(%roomlink(, e)) |
|
I'm not sure what the difference is. I copy and paste this exactly into my session and it returns the object ID of the room to the east, not the vnum.
When you look at room properties in the mapper, the very first tab (room text) shows a number in the lower right corner. This is the same number that shows up when you hover over a room with the mouse. This is the object ID for the room and not what I'm after.
If you go to the "room properties" tab you will see a VNum field. You can script to change this number to match the room number on your mud, which I have done. This is the number I am trying to get for a given exit.
Thanks again. |
|
|
 |
Daern Sorcerer
Joined: 15 Apr 2011 Posts: 809
|
Posted: Mon Nov 18, 2013 1:02 am |
Yeah, I understand, and that's what it gives me. Strange...
|
|
|
 |
charneus Wizard
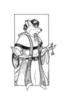
Joined: 19 Jun 2005 Posts: 1876 Location: California
|
Posted: Tue Nov 19, 2013 6:29 am |
Strange, though you can try:
#SHOW %roomvnum(%roomlink(,e))
instead. |
|
|
 |
eupher Apprentice
Joined: 18 Jan 2001 Posts: 116 Location: USA
|
Posted: Tue Nov 19, 2013 1:03 pm |
Even stranger... that returns a number that matches neither the objId OR the vnum. Go figure. :)
|
|
|
 |
Daern Sorcerer
Joined: 15 Apr 2011 Posts: 809
|
Posted: Tue Nov 19, 2013 5:29 pm |
Nah, that shouldn't work, %roomvnum is used to convert filter room numbers (from the %mapfilter function) to keys. %roomnum is the correct function to get the vnum, I don't know why it wouldn't work for you though...
|
|
|
 |
|
|