 |
Xerakon Apprentice
Joined: 10 May 2011 Posts: 111
|
Posted: Thu Jul 05, 2012 11:56 pm
Capturing Information To Place into Interactive Database |
So, here is what I'm looking at. All day long there are communications messages that pop up. IE:
CommNet 0 [A Human Male]: Check.
CommNet 0 [Bob]: Check roger.
CommNet 0 [Bob]: lol
What I want, is a trigger that captures these and places them into a database. It will store them until I erase them by command or whatever. The database sorts the messages by name. IE: A Human Male would have "Check" said at date/time on CommNet 0, and Bob would have the same for "Check roger." and "lol" as two separate entries. Essential to have date/time and where it was said at. I also want to be able to link the person's name, if I see it somewhere, where I can click on it, and brings up their database of messages.
What kind of commands are needed for this?
Thanks! |
|
|
 |
shalimar GURU
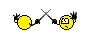
Joined: 04 Aug 2002 Posts: 4691 Location: Pensacola, FL, USA
|
Posted: Fri Jul 06, 2012 12:03 am |
hmm.. you will likely want %time and #ADDKEY and #SHOWDB assuming you will just use a database variable
|
|
_________________ Discord: Shalimarwildcat |
|
|
 |
Rahab Wizard
Joined: 22 Mar 2007 Posts: 2320
|
Posted: Fri Jul 06, 2012 12:29 am |
As an example, if you set up your trigger to capture the name into $name, and the message into $message, you could do something like this:
Code: |
$timedmessage = %concat(%time("hh:mm:ss"), "--", $message)
$currentmessages = %db(@messagelog, $name)
#ADDITEM $currentmessages $timedmessage
#ADDKEY messagelog $name $currentmessages
|
This should end up with something like this:
[A Human Male="15:42:23--Check"|Bob="15:42:30--Check roger.|15:42:35--lol"]
Or you could do something more sophisticated with the time and the message as separate elements which you could use to sort messages by time. |
|
|
 |
Xerakon Apprentice
Joined: 10 May 2011 Posts: 111
|
Posted: Fri Jul 06, 2012 12:36 am |
How much more difficult is that, Rahab? I'm very new to Databases.
|
|
|
 |
Rahab Wizard
Joined: 22 Mar 2007 Posts: 2320
|
Posted: Fri Jul 06, 2012 1:24 am |
Well, a slightly more sophisticated system would be something like:
Code: |
$currentmessages = %db(@messagelog, $name)
$currentmessages = %addkey($currentmessages, {%time("hh:mm:ss"), $message)
#ADDKEY messagelog $name $currentmessages
|
This would produce something like:
[A Human Male="15:42:23=Check"|Bob="15:42:30=Check roger.|15:42:35=lol"]
This would have each time value be the key to the associated message. But this might not be very useful to you, but shows what could be done. Another simple option would be something like:
Code: |
$timedmessage = {}
#ADDKEY $timedmessage time %time("hh:mm:ss")
#ADDKEY $timedmessage message $message
$currentmessages = %db(@messagelog, $name)
#ADDITEM $currentmessages $timedmessage
#ADDKEY messagelog $name $currentmessages
|
This would produce something like (displaying it in perhaps a more understandable way):
Code: |
A Human Male = [
[time=15:42:23|message=Check]
]
Bob = [
[time=15:42:30|message=Check roger.]
[time=15:42:35|message=lol]
]
|
How you format this db variable depends on what you want to do with the data. |
|
|
 |
Xerakon Apprentice
Joined: 10 May 2011 Posts: 111
|
Posted: Fri Jul 06, 2012 1:36 am |
I'm using your second code block, but it's not returning anything
CommNet 0 [A Rodian male]: Sure.
as a sample message
I used
CommNet (%d) [(%d)]: (%d)
as the trigger string to enact the code. Is this correct? |
|
|
 |
Rahab Wizard
Joined: 22 Mar 2007 Posts: 2320
|
Posted: Fri Jul 06, 2012 1:49 am |
No. %d matches a number. So your trigger is trying to match on text like: "CommNet <number> [<number]: <number>". So it isn't matching. Try this pattern:
CommNet (%d) [(*)]: (*) |
|
|
 |
Xerakon Apprentice
Joined: 10 May 2011 Posts: 111
|
Posted: Fri Jul 06, 2012 2:16 am |
Using
Code: |
CommNet (%d) [(*)]: (*)
|
as the pattern, and
Code: |
$timedmessage = {}
#ADDKEY $timedmessage time %time("hh:mm:ss")
#ADDKEY $timedmessage message $message
$currentmessages = %db(@commhist, $name)
#ADDITEM $currentmessages $timedmessage
#ADDKEY messagelog $name $currentmessages
|
as the text....
The pattern does not account for the [] brackets. But even removing the brackets, I get: ERROR: Trigger "CommNet (%d) (*): (*)" fired but did not compile. |
|
|
 |
Rahab Wizard
Joined: 22 Mar 2007 Posts: 2320
|
Posted: Fri Jul 06, 2012 2:37 am |
I didn't give you the entire code. I only gave you a code fragment. It was merely an example of how to do some database variable manipulation. I said that my code depended on you having already set $message and $name. So you need to add some more code. The error message is because you have not defined $message and $name.
|
|
|
 |
Rahab Wizard
Joined: 22 Mar 2007 Posts: 2320
|
Posted: Fri Jul 06, 2012 2:40 am |
As for the brackets, if the pattern is not matching, try quoting the brackets with the ~:
Code: |
CommNet (%d) ~[(*)~]: (*) |
|
|
|
 |
Xerakon Apprentice
Joined: 10 May 2011 Posts: 111
|
Posted: Fri Jul 06, 2012 3:09 am |
Ah, okay. Gotcha. So, here is what I have.
Code: |
CommNet (%d) (*): (*)
$channel = %1
$name = %2
$message = %3
$timedmessage = {}
#ADDKEY $timedmessage channel $channel
#ADDKEY $timedmessage time %time("hh:mm:ss")
#ADDKEY $timedmessage message $message
$currentmessages = %db(@messagelog, $name)
#ADDITEM $currentmessages $timedmessage
#ADDKEY messagelog $name $currentmessages
|
When printed out, it displays
Code: |
[A being in modified katarn-class commando armor]="""channel=2|time=21:59:05|message=test""|""channel=2|time=22:00:43|message=Checking script"""|[Xerakon]="""channel=1|time=22:01:17|message=Also checking"""
|
So, is there a way to break each person and each message apart by a line? Instead of the above, I'd want it to look more like:
Code: |
21:59:05 : Channel 2 : [A being in modified katarn-class commando armor] : test
22:00:43 : Channel 2 : [A being in modified katarn-class commando armor] : Checking script
22:01:17 : Channel 1 : [Xerakon] : Also checking
|
This would be sort of like a traditional log-keeping. Thanks |
|
|
 |
Rahab Wizard
Joined: 22 Mar 2007 Posts: 2320
|
Posted: Fri Jul 06, 2012 1:39 pm |
A database variable is a storage mechanism. It is designed to store information in a particular way. In order to present the data in a readable format, you will need to write a script, probably an alias.
It looks like you actually want this sorted by time, not by name. And do you want the brackets stored in $name? This is why I said that how you format the db variable depends on what you want to do with it. If you can explain exactly what you want to do with the data, we can help you format the variable. Will you actually be using any of the data in any other way? Will you want to be able to limit the display to messages from a particular channel, or a particular time period, or a particular person? Will you want to be able to choose which column to sort by? Will you be using the data in other scripts? You need to think about all this and describe exactly what you want to do before trying to write the script, because all of this affects how you should store the data. For instance, if the only thing you want is to display the entire log in the format you just showed, you don't need a database variable at all--you could simply format each line as it comes in and store it in a stringlist. |
|
|
 |
Xerakon Apprentice
Joined: 10 May 2011 Posts: 111
|
Posted: Fri Jul 06, 2012 2:07 pm |
I would not like brackets to be shown.
I will mostly be using the data to display, but I would also want to use it to spit out to the mud at times (IE, displaying the recorded message back to the MUD incase someone wants to see it. I also will want to make a linker program, where if I see, say, Xerakon talking on the commnet, I can click on the link pulling up on his name, and it will display all of his messages.
As for limitations, I would like to have an alias to put all of the information in the database that pushes it into a historical archive, and wipes the current database clean. There are actually multiple inputs, besides just the commnet channel.
The column should sort by time, but I would assume that when I use the linker program, it will sort by name, then time?
The data would not be used in any scripts other than the display to myself, and the output to the MUD one as mentioned above. |
|
|
 |
Rahab Wizard
Joined: 22 Mar 2007 Posts: 2320
|
Posted: Fri Jul 06, 2012 6:01 pm |
All right. I think the most flexible format would be to have each message be a db string, and have the list of messages be a stringlist of these. It would be structured like this (this is just a diagram of the structure, not how it will actually look):
Code: |
[
[time=15:42:23|channel=0|name=A Human Male|message=Check]
[time=15:42:30|channel=1|name=Bob|message=Check roger.]
[time=15:42:35|channel=1|name=Bob|message=lol]
] |
We can write scripts to grab whatever lines we want from this structure, sort it by whatever columns we like, and format it for display however we like.
The trigger pattern should be:
Code: |
CommNet (%d) ~[(*)~]: (*) |
And the trigger script should be (note that I've changed the %time() output, so that you can store the date for your archive):
Code: |
$channel = %1
$name = %2
$message = %3
$timedmessage = {}
#ADDKEY $timedmessage time %time("yyyy/mm/dd hh:mm:ss")
#ADDKEY $timedmessage channel $channel
#ADDKEY $timedmessage name $name
#ADDKEY $timedmessage message $message
#ADDITEM messagelog $timedmessage
|
Next I'll try to write an alias that will display this the way you want
[edited to fix some of the code] |
|
|
 |
Rahab Wizard
Joined: 22 Mar 2007 Posts: 2320
|
Posted: Fri Jul 06, 2012 6:34 pm |
Here is a quick alias that will display the last 10 messages. This is mostly to show you some of the Cmud features that you can use to do this sort of thing. You should customize this as you wish. Note that I am taking advantage of the fact that items in the stringlist are stored in the order that they are entered, so the stringlist is already in order by time--I don't have to sort it! You can put this in an alias named "recentcomms" or something like that:
Code: |
$numitems = %numitems(@messagelog)
#LOOP %max(($numitems-10),1),$numitems {
$item = %item(@messagelog, %i)
#SHOW %format("&s : Channel &s : &20s : &s", $item.time, $item.channel, $item.name, $item.message)
}
|
And heres a script for an alias to display all the messages from a specific person (again, taking advantage of the fact that the stringlist is in order by time):
Code: |
$wantedname = %1
#FORALL @messagelog {
#IF (%i.name = $wantedname) {#SHOW %format("&s : Channel &s : &20s : &s", %i.time, %i.channel, %i.name, %i.message)}
|
For a script to copy a message to send to someone else, you'll have to explain how you would be sending this to the other person, what format you want it sent in, and how you would identify which message you want sent. |
|
|
 |
Xerakon Apprentice
Joined: 10 May 2011 Posts: 111
|
Posted: Fri Jul 06, 2012 7:35 pm |
What is the trigger pattern for the second code? I've tried something like "recentcommsind (*)" to no avail.
|
|
|
 |
shalimar GURU
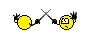
Joined: 04 Aug 2002 Posts: 4691 Location: Pensacola, FL, USA
|
Posted: Fri Jul 06, 2012 8:03 pm |
he said it was an alias, not a trigger, the name doesnt really matter
|
|
_________________ Discord: Shalimarwildcat |
|
|
 |
Rahab Wizard
Joined: 22 Mar 2007 Posts: 2320
|
Posted: Fri Jul 06, 2012 8:06 pm |
That wouldn't be for a trigger. That is intended for an alias. Put that code in an alias named "recentcomms". Then, when you want to look at the last 10 messages, just type
on the command line, and it will print them out for you, nicely formatted. Similarly, the last code block is intended for an alias. Put that into an alias named "commsfrom" or whatever you want. Then, when you type
it will display all the messages from Bob.
|
|
|
 |
Rahab Wizard
Joined: 22 Mar 2007 Posts: 2320
|
Posted: Fri Jul 06, 2012 8:10 pm |
Actually, I just realized that some of the names in your messages are more than one word. On that last code block, change
to
Code: |
$wantedname = %params |
That way it will work when you want to see messages from someone like "A Human Male" |
|
|
 |
Xerakon Apprentice
Joined: 10 May 2011 Posts: 111
|
Posted: Fri Jul 06, 2012 9:02 pm |
Aye, I dunno why I said trigger, it was in an alias.
recentcomms works fine, but the commsfrom only returns "ERROR: Syntax error in Alias: commsfrom : illegal token: " This happens when I use "commsfrom" or "commsfrom name". I did update the $wantedname as well. |
|
|
 |
Daern Sorcerer
Joined: 15 Apr 2011 Posts: 809
|
Posted: Fri Jul 06, 2012 9:18 pm |
There's a missing } to close the #forall.
|
|
|
 |
Xerakon Apprentice
Joined: 10 May 2011 Posts: 111
|
Posted: Fri Jul 06, 2012 10:51 pm |
Okay, added the } to the end of the coding. When I used "commsfrom", it returned
Code: |
2012/07/06 14:32:03 : Channel 2 : Xerakon : Test
|
which is fine, but trying another name, or even Xerakon again after that, it would return nothing. |
|
|
 |
Rahab Wizard
Joined: 22 Mar 2007 Posts: 2320
|
Posted: Sat Jul 07, 2012 5:24 am |
That's odd. That alias does not change the variable at all, so the data is all still there and it should work fine. Are you certain that you spelled it right in the subsequent tests?
|
|
|
 |
Xerakon Apprentice
Joined: 10 May 2011 Posts: 111
|
Posted: Sat Jul 07, 2012 1:33 pm |
I think what happened is that if I spell the name incorrectly in a prior test, it cuts out the loop in all of the following tests. Is there a way to reset it if I spell a name wrong? Thanks!
|
|
|
 |
Rahab Wizard
Joined: 22 Mar 2007 Posts: 2320
|
Posted: Sat Jul 07, 2012 2:51 pm |
That shouldn't affect it, because commsfrom doesn't change the raw data. Could you run 'recentcomms' and then 'commsfrom' using one of the names that shows up in recentcomms, then cut and paste all of that into a post so we can see it happening? [edit]Also, could you cut and paste the XML for your commsfrom alias so we can check the coding?[/edit]
|
|
|
 |
|
|