 |
chamenas Wizard
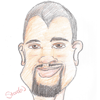
Joined: 26 Mar 2008 Posts: 1547
|
Posted: Sat Mar 20, 2010 5:25 pm
REGEX issue |
So, yet another pattern-matching issue. I've used REGEX coach, but, unfortunately, I'm not sure how to get it to tell me what's wrong. It simply tells me whether oa pattern matches and where it stops matching. In this particular issue, the pattern will match, but one of the values is not being stored when it should.
Here's the REGEX:
Code: |
\[(\d+) (\w+)\] \[ ([\w '-]+) \]\s+([\w'-]+)\s+([\w '-]+(?:\:\w+)?(?: \(\w+\))?)(?:\s+)?(\(Leader\))?(?:\s+)?(\(Recruiter\))?
|
And here's the test phrase:
Code: |
[51 Pri] [ Justice ] Arreana Lieutenant (Survival) (Recruiter)
|
Here's what I expect to get:
%1 = 51
%2 = Pri
%3 = Justice
%4 = Arreana
%5 = Lieutenant (Survival)
Here's what I get:
%1 = 51
%2 = Pri
%3 = Justice
%4 = Arreana
%5 = Lieutenant
Now, if you look closely, you'll see that %5 has some exception clauses, mainly, %5 can either be:
Something
OR
Something:Something
OR
Something (Something)
This phrase:
Code: |
[51 Dru] [ Knighthood ] Branzol Knight:Lance
|
Gives me:
%1 = 51
%2 = Dru
%3 = Knighthood
%4 = Branzol
%5 = Knight:Lance
Exactly what I'm expecting.
If I take out the ? which makes [Something (Something)] optional, then I get my intended %5 = Lieutenant (Survival) but, as you can guess, anything without the " (Something)" no longer matches.
Can anyone see anything that I'm not seeing? |
|
|
 |
charneus Wizard
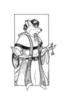
Joined: 19 Jun 2005 Posts: 1876 Location: California
|
Posted: Sat Mar 20, 2010 6:43 pm |
You're not seeing that since it's no longer optional, it's always going to look for that particular phrase. If you're always going to capture the fifth argument, I suggest changing your trigger to this:
Code: |
\[(\d+) (\w+)\] \[ ([\w '-]+) \]\s+([\w'-]+)(?:\s+(.+))?$ |
That way, if there is indeed a fifth argument, it will always capture it, whereas if there isn't, then it'll only capture the first four. If this doesn't do what you're looking for, you're going to need to be a bit more descriptive, provide examples of what you want to catch and what you DON'T want to catch.
Charneus |
|
|
 |
chamenas Wizard
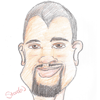
Joined: 26 Mar 2008 Posts: 1547
|
Posted: Sat Mar 20, 2010 7:41 pm |
Yeah, I don't always want to capture the 5th argument. Also, I've noticed that, for some reason, my capture is also capturing spaces sometimes, which is definitely unintended. Here's what I wish to capture:
Code: |
[Lvl Class] [ Clan ] Name Rank (Leader) (Recruiter)
|
Here's the issue, Not everyone has a (Leader) or (Recruiter) flag. Those are both optional. But, if the (Leader) flag is there, I want to capture that value and store it. Incidentally, in case it matters, (Leader) will always be followed by (Recruiter).
The number of spaces between [ Clan ] and Name is variable, hence the \s+
The number of spaces between Name and Rank is variable, hence the \s+
The following is an example who list:
Code: |
[51 Pri] [ Justice ] Arreana Lieutenant (Survival) (Recruiter)
[51 Dru] [ Knighthood ] Branzol Knight:Lance
[51 Inv] [ White Robes ] Ambrosse Advisor (Recruiter)
[51 Cru] [ Knighthood ] Cairhien Knight:Lance
[51 Cru] [ Knighthood ] Thrakhath General (Leader) (Recruiter)
[51 Enc] [ Bloodlust ] Qilue Deathknight (Recruiter)
[51 Pri] [ Bloodlust ] Nyxt Spiritualist (Recruiter)
[51 Mag] [ Black Robes ] Luiz Apprentice
[51 Jng] [ Wargar ] Berhar Oreborn
[51 Asn] [ Bloodlust ] Vandavar Warlord (Leader) (Recruiter)
[51 Nec] [ Bloodlust ] Moran Blood Reaver (Recruiter)
[51 Dra] ( Dragon ) Sylpheed Member
[51 Jng] [ Wargar ] Torral Oreborn
[51 Dru] [ Knighthood ] Henrietta Lieutenant:Shield (Recruiter)
[51 Pal] [ Knighthood ] Gwaine General (Leader) (Recruiter)
[51 Enc] [ Wargar ] Verque Longbeard (Recruiter)
[51 Enc] [ Knighthood ] Anysse General (Leader) (Recruiter)
[51 Cru] [ Knighthood ] Eckert Page
[51 Run] [ Wargar ] Betha Thane (Leader) (Recruiter)
[51 Cru] [ Wargar ] Dorigar Clansmen
[51 Eld] [ Shalonesti ] Amyth'lynn Keeper
[51 Skd] [ Slayers ] Chucco Follower
[49 Jng] [ Knighthood ] Dredo Page
[47 Nsh] [ Slayers ] Diverion Unproven
[47 Swb] [ Knighthood ] Tryiteryn Page
[37 Bat] [ Wargar ] Iulh Clansmen
[24 Mag] [ Red Robes ] Bra'hvchk Student
[ 5 Mag] [ Red Robes ] Dxutim Student
[ 5 Dra] ( Dragon ) Dresuril Member
|
Anything that has ( Dragon ) I'm not worried about, hence why it isn't considered. There is an interesting issue with any single-digit levels, since it adds a space for formatting, but that doesn't worry me as most people won't clan until 25. (Clans have the [ ] around them and are the PK segment of the MUD. You can also be in a Kingdom, but I'm not worried about Kingdomers).
As you can see, every value will always have
Code: |
[Lvl Class] [ Clan ] Name Rank
|
So that's a given. The issue is that, sometimes rank can be:
Rank
Sometimes it's
Rank:Sub
Sometimes it's
Rank (Sub)
The trickiest issue in this is the Rank (Sub) since (Sub) is similar to (Leader), and it's possible to have:
Rank (Sub) (Recruiter)
like you can see here:
Code: |
51 Pri] [ Justice ] Arreana Lieutenant (Survival) (Recruiter)
|
Survival is part of Rank, and I'd like it stored as part of Rank.
As far as spaces being captured...
Code: |
[51 Cru] [ Knighthood ] Eckert Page < Line ends before this arrow
|
Sometimes the line ends way after the Rank. I don't want to save those spaces, but sometimes the Rank is two words, such as with:
Code: |
[51 Skd] [ Justice ] Qzxauip Lord of War (Leader) (Recruiter)
|
Luckily, multiple words in the Rank is a rare exception, so I could possibly store all of those in a variable and add them as a contingent exception? (Check to see if Rank = Variable List, if not, get the one-word Rank) ? |
|
|
 |
charneus Wizard
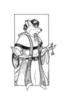
Joined: 19 Jun 2005 Posts: 1876 Location: California
|
Posted: Sat Mar 20, 2010 8:10 pm |
Wait, are you only wanting to capture (lLeader) (Recruiter), or are you wanting to capture anything that has a rank? You're not being completely specific about /what/ you want to capture. Out of that who list you showed, show me what you're actually capturing (or wishing to, rather).
Charneus |
|
|
 |
charneus Wizard
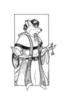
Joined: 19 Jun 2005 Posts: 1876 Location: California
|
Posted: Sat Mar 20, 2010 8:22 pm |
Code: |
^\[\s?(\d+) (\w{3})\] \[ ([\w '-]+) \]\s+([\w '-]+)\s+ (\w+(?: \w+)?:?(?:\s+)?\(?\w+?\)?\s+\(?(?:\w+)?\)?) |
Out of your who list, it matches everything that is not ( Dragon ). It stores the rank as %5 correctly (as far as I can see) and does what you want. You /can/ do an #IF (%5) check in the trigger as well. If %5 is there, it'll return true. If it's not, it'll return false. As for extra spaces, you can always remove them in the trigger with %trim or %replace.
Charneus |
|
|
 |
chamenas Wizard
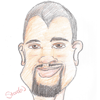
Joined: 26 Mar 2008 Posts: 1547
|
Posted: Sat Mar 20, 2010 8:35 pm |
In response to your question, I wish to capture their Level, Class, Clan, Name and Rank. Everyone will have those values.
(Leader) and (Recruiter) are flags that only SOME people will have. However, of those, I wish to capture the (Leader) flag.
Anyone who has a (Leader) flag will also have a (Recruiter) flag, but the (Recruiter) flag is inconsequential, I don't care about it.
There are some people who have the (Recruiter) flag but NOT the (Leader) flag. In this case, I only want to capture Level, Class, Clan, Name, and Rank. I don't want to capture any flags.
Thanks for the responses, I will test them out and get back to you.
Edit: Tested
Yeah, I apologize. I thought I had been clear, but apparently I hadn't.
%1 = Level
%2 = Class
%3 = Clan
%4 = Name
%5 = Rank
%6 = Leader (if it doesn't exist, %6 should be = %null)
Here's the WHOLE trigger. Right now, the only thing of consequence is the pattern. Hence why I didn't share it, but if you feel it will help you gain a better understanding of what's wanted...
Code: |
<trigger name="Gather-Clanners" priority="3660" regex="true" enabled="false" id="366">
<pattern>^\[\s?(\d+) (\w{3})\] \[ ([\w '-]+) \]\s+([\w '-]+)\s+ (\w+(?: \w+)?:?(?:\s+)?\(?\w+?\)?\s+\(?(?:\w+)?\)?)</pattern>
<value>#if (%ismember(%4, @c_name))
{
$_member_num = %ismember(%4, @c_name)
$_curr_lvl = %item(@c_lvl, $_member_num)
$_curr_class = %item(@c_class, $_member_num)
$_curr_clan = %item(@c_clan, $_member_num)
$_curr_rank = %item(@c_rank, $_member_num)
$_curr_is_leader = %item(@c_leader, $_member_num)
#if ($_curr_lvl != %1)
{
$_old_lvl = $_curr_lvl
#VAR c_lvl %replaceitem(%1, $_member_num, @c_lvl)
$_new_lvl = %item(@c_lvl, $_member_num)
#show "Level changed from "$_old_lvl" to "$_new_lvl" for: "%4
}
$_sel_class = "NULL"
#switch (%2)
("War") {$_sel_class = "Warrior"}
("Ran") {$_sel_class = "Ranger"}
("Bar") {$_sel_class = "Barbarian"}
("Swb") {$_sel_class = "Swashbuckler"}
("Arm") {$_sel_class = "Armsman"}
("Cle") {$_sel_class = "Cleric"}
("Cru") {$_sel_class = "Crusader"}
("Dru") {$_sel_class = "Druid"}
("Sha") {$_sel_class = "Shaman"}
("Pri") {$_sel_class = "Priest"}
("Mag") {$_sel_class = "Mage"}
("Wit") {$_sel_class = "Witch"}
("Wlk") {$_sel_class = "Warlock"}
("Ill") {$_sel_class = "Illusionist"}
("Enc") {$_sel_class = "Enchantor"}
("Brd") {$_sel_class = "Bard"}
("Jng") {$_sel_class = "Jongleur"}
("Cha") {$_sel_class = "Charlatan"}
("Skd") {$_sel_class = "Skald"}
("Thi") {$_sel_class = "Thief"}
("Asn") {$_sel_class = "Assassin"}
("Bnd") {$_sel_class = "Bandit"}
("Nsh") {$_sel_class = "Nightshade"}
("Inv") {$_sel_class = "Invoker"}
("Tra") {$_sel_class = "Transmuter"}
("Bmg") {$_sel_class = "Battlemage"}
("Nec") {$_sel_class = "Necromancer"}
("Pal") {$_sel_class = "Paladin"}
("Skn") {$_sel_class = "Shadowknight"}
("Bla") {$_sel_class = "Bladesinger"}
("Eld") {$_sel_class = "Eldritch"}
("Bat") {$_sel_class = "Battlerager"}
("Run") {$_sel_class = "Runesmith"}
("Mon") {$_sel_class = "Monk"}
{#show "No class value found"}
#if ($_sel_class != $_curr_class)
{
$_old_class = $_curr_class
#VAR c_class %replaceitem($_sel_class, $_member_num, @c_class)
$_new_class = %item(@c_class, $_member_num)
#show "Class changed from "$_old_class" to "$_new_class" for: "%4
}
#if ($_curr_clan != %3)
{
$_old_clan = $_curr_clan
#VAR c_clan %replaceitem(%3, $_member_num, @c_clan)
$_new_clan = %item(@c_clan, $_member_num)
#show "Clan changed from "$_old_clan" to "$_new_clan" for: "%4
}
#if ($_curr_rank != %5)
{
$_old_rank = $_curr_rank
#VAR c_rank %replaceitem(%5, $_member_num, @c_rank)
$_new_rank = %item(@c_rank, $_member_num)
#show "Rank changed from "$_old_rank" to "$_new_rank" for: "%4
}
#if (%6 != %null)
{
$_is_leader = "TRUE"
#if ($_is_leader != $_curr_is_leader)
{
$_old_leader = $_curr_is_leader
#VAR c_leader %replaceitem(%6, $_member_num, @c_leader)
$_new_leader = %item(@c_leader, $_member_num)
#show "Leader changed from "$_old_leader" to "$_new_leader" for: "%4
}
}
}
{
#show "Adding a new character... "%4
#ADDI c_name %4
#VAR c_lvl %additem(%1, @c_lvl)
#switch (%2)
("War") {#VAR c_class %additem("Warrior", @c_class)}
("Ran") {#VAR c_class %additem("Ranger", @c_class)}
("Bar") {#VAR c_class %additem("Barbarian", @c_class)}
("Swb") {#VAR c_class %additem("Swashbuckler", @c_class)}
("Arm") {#VAR c_class %additem("Armsman", @c_class)}
("Cle") {#VAR c_class %additem("Cleric", @c_class)}
("Cru") {#VAR c_class %additem("Crusader", @c_class)}
("Dru") {#VAR c_class %additem("Druid", @c_class)}
("Sha") {#VAR c_class %additem("Shaman", @c_class)}
("Pri") {#VAR c_class %additem("Priest", @c_class)}
("Mag") {#VAR c_class %additem("Mage", @c_class)}
("Wit") {#VAR c_class %additem("Witch", @c_class)}
("Wlk") {#VAR c_class %additem("Warlock", @c_class)}
("Ill") {#VAR c_class %additem("Illusionist", @c_class)}
("Enc") {#VAR c_class %additem("Enchantor", @c_class)}
("Brd") {#VAR c_class %additem("Bard", @c_class)}
("Jng") {#VAR c_class %additem("Jongleur", @c_class)}
("Cha") {#VAR c_class %additem("Charlatan", @c_class)}
("Skd") {#VAR c_class %additem("Skald", @c_class)}
("Thi") {#VAR c_class %additem("Thief", @c_class)}
("Asn") {#VAR c_class %additem("Assassin", @c_class)}
("Bnd") {#VAR c_class %additem("Bandit", @c_class)}
("Nsh") {#VAR c_class %additem("Nightshade", @c_class)}
("Inv") {#VAR c_class %additem("Invoker", @c_class)}
("Tra") {#VAR c_class %additem("Transmuter", @c_class)}
("Bmg") {#VAR c_class %additem("Battlemage", @c_class)}
("Nec") {#VAR c_class %additem("Necromancer", @c_class)}
("Pal") {#VAR c_class %additem("Paladin", @c_class)}
("Skn") {#VAR c_class %additem("Shadowknight", @c_class)}
("Bla") {#VAR c_class %additem("Bladesinger", @c_class)}
("Eld") {#VAR c_class %additem("Eldritch", @c_class)}
("Bat") {#VAR c_class %additem("Battlerager", @c_class)}
("Run") {#VAR c_class %additem("Runesmith", @c_class)}
("Mon") {#VAR c_class %additem("Monk", @c_class)}
{#show "No class value found"}
#VAR c_clan %additem(%3, @c_clan)
#VAR c_rank %additem(%5, @c_rank)
#if (%6 != %null)
{
#VAR c_leader %additem("TRUE", @c_leader)
}
{
#VAR c_leader %additem("FALSE", @c_leader)
}
$_new_member = %ismember(%4, c_name)
}</value>
</trigger>
|
|
|
_________________ Listen to my Guitar - If you like it, listen to more
Last edited by chamenas on Sat Mar 20, 2010 8:40 pm; edited 1 time in total |
|
|
 |
charneus Wizard
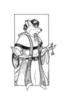
Joined: 19 Jun 2005 Posts: 1876 Location: California
|
Posted: Sat Mar 20, 2010 8:39 pm |
Okay, so the (Leader) (Recruiter) or (Survival) (Recruiter) or even just (Recruiter) -- those are the flags you're talking about, and you only want to store the flags with the rank if it's a (Leader) only? Is that what you're saying? Or are you wanting to actually store flags as a 6th argument?
Charneus |
|
|
 |
chamenas Wizard
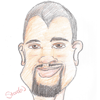
Joined: 26 Mar 2008 Posts: 1547
|
Posted: Sat Mar 20, 2010 8:47 pm |
(Survival) isn't actually a flag, it's part of Rank, it just looks like a flag because of the (), but should be stored with Rank, i.e:
%5 (Rank) = Lieutenant (Survival)
%6 is for the (Leader) flag only. The (Recruiter) will be in the line sometimes, but I don't want to store it in %6. If there is no (Leader) then %6 will be null. It will end up being null most of the time.
Edit: Also, I edited the post above with the results of the test. If the pattern in the copied and pasted trigger doesn't match yours, it's because I pasted my old one back in for the time being, but I did in fact test yours. |
|
|
 |
charneus Wizard
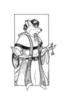
Joined: 19 Jun 2005 Posts: 1876 Location: California
|
Posted: Sat Mar 20, 2010 8:53 pm |
Okay, perhaps this is what you're looking for, then:
Code: |
^\[\s?(\d+) (\w{3})\] \[ ([\w '-]+) \]\s+([\w'-]+)\s+([\w '-]+):?(?:\s+)?(?:\((Leader)\))? |
It'll match a line whether it contains (Leader) or not, and if it does contain Leader, it's stored as the 6th argument. Unless I'm missing anything else, this should work for you perfectly.
Charneus |
|
|
 |
chamenas Wizard
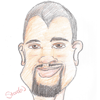
Joined: 26 Mar 2008 Posts: 1547
|
Posted: Sat Mar 20, 2010 9:01 pm |
What you've given me is slightly different (presumably more efficient) than mine, but it still has the same error with:
Code: |
[51 Pri] [ Justice ] Arreana Lieutenant (Survival) (Recruiter)
|
%5 = Lieutenant
when it should be
%5 = Lieutenant (Survival)
It actually also captures
Code: |
[51 Dru] [ Knighthood ] Branzol Knight:Lance
|
%5 = Knight:
instead of
%5 = Knight:Lance
It's so very close, and yet not precise. Perhaps you can see why it's giving me a headache?  |
|
|
 |
charneus Wizard
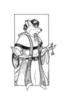
Joined: 19 Jun 2005 Posts: 1876 Location: California
|
Posted: Sat Mar 20, 2010 9:48 pm |
Assuming (Survival) is the only 'rank addition, use this:
Code: |
^\[\s?(\d+) (\w{3})\] \[ ([\w '-]+) \]\s+([\w'-]+)\s+([\w '-]+:?(?:\s+)?(?:\(Survival\)|\w+)?)(?:\s+)?(?:\((Leader)\))? |
It wasn't giving me a headache at all. You were just poorly explaining what you wanted to have done. :\
Charneus |
|
|
 |
chamenas Wizard
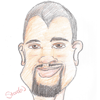
Joined: 26 Mar 2008 Posts: 1547
|
Posted: Sat Mar 20, 2010 9:55 pm |
Survival isn't the only rank addition, I just can't think of the others currently. However, I assume I can sub the word "Survival" with a more general statement? There's also the issue with any rank that comes after : as in:
Knight:Lance
Edit: Bleh, I didn't mean to come off as attacking. Just felt insulted, apologies.
Also, while changing Survival to \w+ worked marvelously, it, as you probably could have told me, added flags to people's ranks if the flag existed. Gaaaah. |
|
|
 |
chamenas Wizard
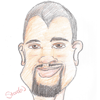
Joined: 26 Mar 2008 Posts: 1547
|
Posted: Sat Mar 20, 2010 10:06 pm |
Perhaps I should simply keep the pattern as I have it now, with your suggestion and my \w+ in place of survival and simply have code in the trigger that searches ranks for flags, notes them and trims them out?
|
|
|
 |
charneus Wizard
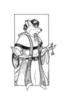
Joined: 19 Jun 2005 Posts: 1876 Location: California
|
Posted: Sat Mar 20, 2010 10:13 pm |
The issue with any rank that comes after the : like in Knight:Lance was solved with my last regex.
As for a more general statement for other rank additions, your best bet is to create a list of rank additions and use that variable in place of the Survival, so it'd look like this:
(?:@rankadditions)
instead, or whatever variable you choose to use.
Charneus |
|
|
 |
chamenas Wizard
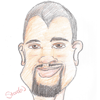
Joined: 26 Mar 2008 Posts: 1547
|
Posted: Sat Mar 20, 2010 10:25 pm |
I decided to forgo the leader stuff, and simply have the flags trimmed out of rank. It works perfectly and I understand the differences. Thank you, and I do apologize that I couldn't communicate it clearly. Not for lack of trying though!
|
|
|
 |
|
|
|
You cannot post new topics in this forum You cannot reply to topics in this forum You cannot edit your posts in this forum You cannot delete your posts in this forum You cannot vote in polls in this forum
|
|