 |
chamenas Wizard
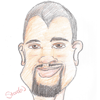
Joined: 26 Mar 2008 Posts: 1547
|
Posted: Thu Feb 05, 2009 3:46 pm
Matching multiple cases [Solved] |
I'm making a spellbot trigger for people to use when I'm sort of idlish. However, there are a few spells I don't want them to use. Since my MUD, ever efficient, allows you to use partial names, I have to cover all instances of a spell name. For instance, a spell like permanency can be cast as:
c 'perm'
c 'permanency'
c 'perman'
etc...
I was wondering if there was a simple way to cover all cases in a single statement. As you can see, currently, in my trigger, I'm trying to cover all of them through OR statements which is horridly long, etc...
Also, for some reason, when it sends regen (which is an alias) I get "huh" from the MUD, so it's apparently not sending it as an alias.
Code: |
<trigger name="Spellbot" priority="1910" regex="true" id="94">
<pattern>^(?:\(Imm\) )?(?:The ghost of )?([\w']+|A masked swashbuckler|\(An Imm\)){1,2} tells you (?:\([^\)]+\) )?'(cc:(?: )?(.*))'</pattern>
<value>#switch ((%3="perm") OR (%3="per") OR (%3="permanency") OR (%3="perman") OR (%3="perman") OR (%3="permanen") OR (%3="permanenc")) {
#send {"tell "%1" Not on your life. Try another."}
}
(%3="spellup") {
$cur_posn=@posn
#send {"stand"}
#send {"c 'armor' "%1}
#send {"c 'shield' "%1}
#send {"c 'invis' "%1}
#send {"c 'sanctuary' "%1}
#send {$cur_posn @bed}
#send {regen}
}
((%3="chain") OR (%3="chain lightning") OR (%3="chain light") OR (%3="chain li") OR (%3="chain ligh") OR (%3="chain lightni"))
{
#send {"tell "%1" I don't go for masochism, try something else"}
}
(%3="request") {
#send {"tell "%1" use cc: spellname for an ordinary spell. Use cc: spellup to receive armor, shield, invisibility and sanctuary all at once."}
}
{
$cur_posn=@posn
#send {"stand"}
#send {"c '"%3"' "%1}
#send {$cur_posn @bed}
regen
}
</value>
</trigger>
|
|
|
_________________ Listen to my Guitar - If you like it, listen to more
Last edited by chamenas on Thu Feb 05, 2009 10:54 pm; edited 3 times in total |
|
|
 |
Fang Xianfu GURU
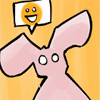
Joined: 26 Jan 2004 Posts: 5155 Location: United Kingdom
|
Posted: Thu Feb 05, 2009 5:42 pm |
Use the %begins function. You can see if the spell they've requested begins any of the names you don't want, and reject if so.
|
|
|
 |
chamenas Wizard
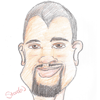
Joined: 26 Mar 2008 Posts: 1547
|
Posted: Thu Feb 05, 2009 7:29 pm |
So if I use begins and permanency, any thing leading up to the word permanency will fail?
Perm
Per
Perman
etc..? |
|
|
 |
Fang Xianfu GURU
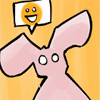
Joined: 26 Jan 2004 Posts: 5155 Location: United Kingdom
|
Posted: Thu Feb 05, 2009 7:49 pm |
#if (!%begins("permanency",%1)) {}
|
|
|
 |
chamenas Wizard
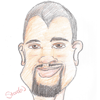
Joined: 26 Mar 2008 Posts: 1547
|
Posted: Thu Feb 05, 2009 8:20 pm |
Thanks, it's going into a switch. By the way 
|
|
|
 |
Fang Xianfu GURU
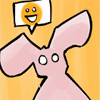
Joined: 26 Jan 2004 Posts: 5155 Location: United Kingdom
|
Posted: Thu Feb 05, 2009 9:12 pm |
Don't use a switch unless you need a different exception for each invalid spell (you probably don't).
#if (%begins("permanency",%1) OR %begins("blahblah",%1)) {invalid} {valid} |
|
|
 |
chamenas Wizard
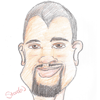
Joined: 26 Mar 2008 Posts: 1547
|
Posted: Thu Feb 05, 2009 9:27 pm |
Alright, I suppose I'll fix that. Then see if I get the same error I was about to report.
|
|
|
 |
chamenas Wizard
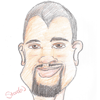
Joined: 26 Mar 2008 Posts: 1547
|
Posted: Thu Feb 05, 2009 9:36 pm |
Here's the new trigger, both sanc, sanctuary brought up the fail message, as well as shield...
Code: |
<trigger name="Spellbot" priority="1910" regex="true" id="94">
<pattern>^(?:\(Imm\) )?(?:The ghost of )?([\w']+|A masked swashbuckler|\(An Imm\)){1,2} tells you (?:\([^\)]+\) )?'(cc:(?: )?(.*))'</pattern>
<value>#IF ((!%begins("permanency",%3)) OR (!%begins("chain lightning", %3)) OR (!%begins("fireball", %3)) OR (!%begins("disjunction", %3)) OR (!%begins("acid blast", %3)) OR (!%begins("blizzra", %3)) OR (!%begins("disjunction", %3)) OR (!%begins("acid blast", %3)) OR (!%begins("blizzra", %3)))
{
#send {"reply Not on your life, try another. (ooc: Automated response to spellbot request)"}
}
{
$cur_posn=@posn
#send {"stand"}
#send {"c '"%3"' "%1}
#send {$cur_posn @bed}
regen
}
</value>
</trigger>
|
|
|
|
 |
Vijilante SubAdmin

Joined: 18 Nov 2001 Posts: 5182
|
Posted: Thu Feb 05, 2009 9:48 pm |
Reverse matching seems a little bit easier.
#IF (%regex("permanency chain lightning fireball disjunction acid blast blizzra",%concat("\b",%3))) |
|
_________________ The only good questions are the ones we have never answered before.
Search the Forums |
|
|
 |
chamenas Wizard
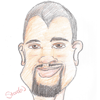
Joined: 26 Mar 2008 Posts: 1547
|
Posted: Thu Feb 05, 2009 9:55 pm |
I'm confused, what does that do exactly?
|
|
|
 |
Fang Xianfu GURU
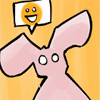
Joined: 26 Jan 2004 Posts: 5155 Location: United Kingdom
|
Posted: Thu Feb 05, 2009 10:23 pm |
It creates a regex pattern "\bpermanency" or whatever and then looks for it in the string that Viji's written. Pretty nifty way of handling it, actually.
However, the problem with this code is simply that you have the true-command and false-command backwards. Compare the code in my two posts above.
Easier (and probably faster) would be to just remove the !s, like I did in the second post. |
|
|
 |
chamenas Wizard
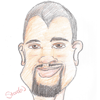
Joined: 26 Mar 2008 Posts: 1547
|
Posted: Fri Feb 06, 2009 12:33 am |
I wonder if using a string list for the spells and putting it in the begins function would work?
|
|
|
 |
gamma_ray Magician
Joined: 17 Apr 2005 Posts: 496
|
Posted: Fri Feb 06, 2009 1:43 am |
No, but you could use a #for @stringlist construction.
|
|
|
 |
chamenas Wizard
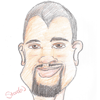
Joined: 26 Mar 2008 Posts: 1547
|
Posted: Fri Feb 06, 2009 2:09 am |
Well, it's not so much a stringlist construction that I want. It's just that there are a lot of spells an thus a lot of exceptions.
|
|
|
 |
Fang Xianfu GURU
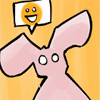
Joined: 26 Jan 2004 Posts: 5155 Location: United Kingdom
|
Posted: Fri Feb 06, 2009 3:03 am |
Use Viji's reverse regex method, then.
|
|
|
 |
chamenas Wizard
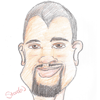
Joined: 26 Mar 2008 Posts: 1547
|
Posted: Fri Feb 06, 2009 3:25 am |
It should work just as well?
|
|
|
 |
|
|