 |
Arde Enchanter
Joined: 09 Sep 2007 Posts: 605
|
Posted: Mon Jan 12, 2009 10:14 pm
Is there any way to preserve Lua table across function calls? |
Say, if I have several functions in settings written in Lua, is there any way to preserve Lua table across their calls?
Thanks.
UPDATE Or may be I can pass a table somehow from 1 function to another? Will CMUD interfere and check what parameter type has been passed from one lua function to another? |
|
|
 |
Fang Xianfu GURU
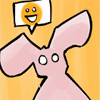
Joined: 26 Jan 2004 Posts: 5155 Location: United Kingdom
|
Posted: Tue Jan 13, 2009 3:37 am |
Er... depends exactly what you're doing as to whether you can "pass a table" to one function or another, but the short answer is yes, you just add a parameter to the function that accepts a table:
function First (someTable)
table.insert(someTable,"lol")
return someTable
end
function Second (aTable)
local bTable = First(aTable)
print(table.concat(bTable))
end
The function Second calls the function First, passing its aTable parameter. The aTable parameter becomes First's someTable param, which is then worked on and returned, being stored in the bTable variable, which is then worked on again. I'm not sure if the First function will actually change the value of aTable or not, it might do - worth checking.
But yeah, you don't need to go to all these elaborate lengths unless there's a specific reason for you to. CMUD uses a single global namespace for all its Lua variables, so if you do a="b" in one setting, a will still equal b in every other setting until it's changed. Simple example:
Code: |
<alias name="one" language="Lua" copy="yes">
<value>a="b"</value>
</alias>
<alias name="two" language="Lua" copy="yes">
<value>print(a)</value>
</alias> |
|
|
|
 |
Arde Enchanter
Joined: 09 Sep 2007 Posts: 605
|
Posted: Tue Jan 13, 2009 6:31 am |
Argh! I've defined test variable as local and obviously for that reason it did not work in my own similar "a='b' / print (a)" test.
Fang Xianfu wrote: |
CMUD uses a single global namespace for all its Lua variables |
That's resolve all my problems with passing a parameter. Thanks! |
|
|
 |
Arde Enchanter
Joined: 09 Sep 2007 Posts: 605
|
Posted: Tue Jan 13, 2009 4:34 pm |
Fang Xianfu wrote: |
function First (someTable)
table.insert(someTable,"lol")
return someTable
end
function Second (aTable)
local bTable = First(aTable)
print(table.concat(bTable))
end
|
This is doable in Lua in general, but is it doable in Lua in CMUD? CMUD has another code organization. Am I right that in CMUD only CMUD functions written in Lua are
available, while in your example you showed Lua functions written in Lua? |
|
|
 |
Tech GURU
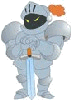
Joined: 18 Oct 2000 Posts: 2733 Location: Atlanta, USA
|
Posted: Tue Jan 13, 2009 4:57 pm |
You can write Lua functions in Lua and have them available withing Lua code contexts. If you want them available to CMUD you'd need a wrapper CMUD function. In other words define a CMUD function with a lua script type that returns the value of the Lua function you want to call.
|
|
_________________ Asati di tempari! |
|
|
 |
Arde Enchanter
Joined: 09 Sep 2007 Posts: 605
|
Posted: Tue Jan 13, 2009 5:07 pm |
Tech wrote: |
You can write Lua functions in Lua and have them available withing Lua code contexts. |
Ok, could you then give me step-by-step instructions how I can do this? I need technical information, generally "where I must enter my code". |
|
|
 |
Fang Xianfu GURU
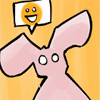
Joined: 26 Jan 2004 Posts: 5155 Location: United Kingdom
|
Posted: Tue Jan 13, 2009 6:19 pm |
Lua functions are just another value for Lua variables - you just put your function-defining code anywhere in CMUD where it'll be run, and then run it (an OnConnect event works best). After that, whatever variable you put your function in will have that function in everywhere.
|
|
|
 |
Tech GURU
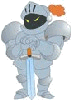
Joined: 18 Oct 2000 Posts: 2733 Location: Atlanta, USA
|
Posted: Tue Jan 13, 2009 6:45 pm |
Here's an example from my code.
Code: |
<?xml version="1.0" encoding="ISO-8859-1" ?>
<cmud>
<func name="addOwner" copy="yes">
<value>function addOwner(owner, mud, description)
if (conn == nil) then
return nil;
else
insOwner = { "INSERT INTO owner (name,mud,description) VALUES(", nil,"','", nil ,"','", nil,")"}
insOwner[2] = owner;
insOwner[4] = mud;
insOwner[6] = description;
query = "";
for i,v in pairs(insStock) do
query = query .. v;
end
debug (query)
cur = conn:execute( query )
return (true)
end
end
debug (zs.param(0))
return addOwner(zs.param(1), zs.param(2), zs.param(3));
</value>
<arglist>$owner,$mud,$description</arglist>
</func>
</cmud> |
|
|
_________________ Asati di tempari! |
|
|
 |
Fang Xianfu GURU
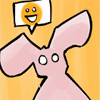
Joined: 26 Jan 2004 Posts: 5155 Location: United Kingdom
|
Posted: Tue Jan 13, 2009 6:56 pm |
Actually, doing the function...end every time wastes valuable time, but putting the function code inside the function itself is a great way of doing it. If it were me, I'd do it something like
if not AddOwner then
function AddOwner ... end
end
debug (zs.param(0))
return addOwner(zs.param(1), zs.param(2), zs.param(3))
What I meant was more like putting the function...end part anywhere you like, in an OnConnect event or something you know will have run before you try to call this function (because trying to call an undefined function will throw an error). Then you just call the function as you're doing in the last line, any time you want.
The if not addOwner method will define the function the first time it's run, but after that it'll be skipped. |
|
|
 |
Arde Enchanter
Joined: 09 Sep 2007 Posts: 605
|
Posted: Tue Jan 13, 2009 7:25 pm |
Fang Xianfu:
Sorry, but it was hard for me to understand what did you say about functions in variable until I see Tech' example.
Tech:
Thank you! I think it is the second example of a real Lua function in the whole forum (first post was made by Vijilante some time ago).
So... Start point here is debug (zs.param(0)) call...
Will CMUD accept if I'll add more than 1 function definition? I need a helper function with var args defined as well as primary. |
|
_________________ My personal bug|wish list:
-Wrong Priority when copy-paste setting
-1 prompt trigger for Mapper, Session and General Options, not 3 different!
-#SECTION can terminate threads
-Buttons can't start threads |
|
|
 |
Tech GURU
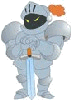
Joined: 18 Oct 2000 Posts: 2733 Location: Atlanta, USA
|
Posted: Tue Jan 13, 2009 9:15 pm |
Yeah Fang.. I realized it's not optimized. It's some code I pulled from something in progress (and actually on the back burner right now) so wasn't very organized, but it was a ready example I had available.
|
|
_________________ Asati di tempari! |
|
|
 |
Arde Enchanter
Joined: 09 Sep 2007 Posts: 605
|
Posted: Mon Jan 19, 2009 10:24 pm |
Heh, now I have another question. It seems that Lua context get saved across closed sessions. I use a package that I open manually in the untitled session. If after Lua function initialization I make a change in its code, choose "Close All" and in the new untitled session open my package again, CMUD executes the old version of that function. Is it intentional? Or by choosing "Close All" CMUD does not actually create the new untitled session?
|
|
_________________ My personal bug|wish list:
-Wrong Priority when copy-paste setting
-1 prompt trigger for Mapper, Session and General Options, not 3 different!
-#SECTION can terminate threads
-Buttons can't start threads |
|
|
 |
Fang Xianfu GURU
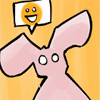
Joined: 26 Jan 2004 Posts: 5155 Location: United Kingdom
|
Posted: Tue Jan 20, 2009 6:32 am |
I suspect that this happens because the Lua namespace is held separate from CMUD - it'll have the same contents until you close CMUD and Lua is shut down entirely.
|
|
|
 |
Vijilante SubAdmin

Joined: 18 Nov 2001 Posts: 5182
|
Posted: Tue Jan 20, 2009 11:58 pm |
Depending on how Zugg set up the loading for Lua it should be possible to flush all Lua data with each session. I suspect Zugg did set it up in such a fashion that moving a few lines of code around is about all it would take to make Lua flush when a session is closed, and load clean again with the new session.
|
|
_________________ The only good questions are the ones we have never answered before.
Search the Forums |
|
|
 |
Fang Xianfu GURU
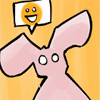
Joined: 26 Jan 2004 Posts: 5155 Location: United Kingdom
|
Posted: Wed Jan 21, 2009 8:36 am |
If it were me, I'd be storing all my functions and variables in separate tables, to keep them organised into modules. My notes system has a table, my curing system has a table, my balance-tracking has a table, and so on. That way you can just set those tables back to nil and everything is gone.
You could also just do if SomeFunc then SomeFunc=nil end before each definition, if you want to make sure it's an updated version. |
|
|
 |
|
|