 |
charneus Wizard
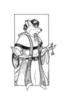
Joined: 19 Jun 2005 Posts: 1876 Location: California
|
Posted: Mon May 05, 2008 7:28 pm
REGEX question |
I have a workaround for what I'm trying to accomplish, but I'm trying to see if a REGEX would make things a bit easier. I got some help on this, but of course, it keeps firing because the pattern matches every time. *sigh*
Ok. Currently, there are about 25 different combinations of our shortflags, which are (K), (I), (M), (G), (H). Retaining order, they can be any combination of those flags, so it could be (K)(I)(M), but not (K)(M)(I). I used a database variable, setting (K)(I)(M) to equal (KIM), and triggered off the key and subbed it with the value. Of course, this only worked for zMUD. I'm still trying to get it to work in CMUD.
Anyway, the REGEX I was trying was:
#REGEX {([KIMGH()]+)} {$flagsub=%replace(%1,")");$flagsub=%replace(%1,"(");#SUB {~($flagsub~)}}
So, what can I do to remedy this? Or are databases probably the easiest way?
Charneus |
|
|
 |
Larkin Wizard

Joined: 25 Mar 2003 Posts: 1113 Location: USA
|
Posted: Mon May 05, 2008 7:32 pm |
How about something like this for your pattern?
|
|
|
 |
charneus Wizard
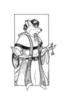
Joined: 19 Jun 2005 Posts: 1876 Location: California
|
Posted: Mon May 05, 2008 7:45 pm |
Well, could you explain how that would work? I'd need it to capture (K), (I), (M), (G), (H), (K)(I), (K)(M), (K)(G), etc...
So basically, the text received vs. the output would look like this:
(K)(I)(M)(G)(H) -> (KIMGH)
(K)(M)(G) -> (KMG) and so forth.
This is driving me insane. :P
Charneus |
|
|
 |
Larkin Wizard

Joined: 25 Mar 2003 Posts: 1113 Location: USA
|
Posted: Mon May 05, 2008 8:00 pm |
I created a group to put parentheses around any of the letters received and put the letters inside as the optional group inside the square brackets. So, it'll capture just like you said. You can then just use a %subregex to remove the extra parentheses to get the output you want.
To put it all together:
Code: |
#REGEX {((?:\([KIMGH]\))+)} {#SUB %concat("(", %subregex(%1, "[\(\)]", ""), ")")} |
|
|
|
 |
Vijilante SubAdmin

Joined: 18 Nov 2001 Posts: 5182
|
Posted: Mon May 05, 2008 8:08 pm |
I would suggest %subchar as more effiecient for removing the parenthesis.
Code: |
#REGEX {((?:\([KIMGH]\))+)} {#SUB %concat("(", %subchar(%1, "()", ""), ")")} |
|
|
_________________ The only good questions are the ones we have never answered before.
Search the Forums |
|
|
 |
Fang Xianfu GURU
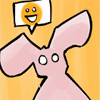
Joined: 26 Jan 2004 Posts: 5155 Location: United Kingdom
|
Posted: Mon May 05, 2008 8:13 pm |
I'd do this like so:
#trig {(\(K\))?(\(I\))?(\(M\))?(\(G\))?(\(H\))?} {#var flags %concat("(",%1,%2,%3,%4,%5,")")}
The regex looks a lot uglier without syntax highlighting, but it looks alright once it's in a trigger. And the script is a piece of piss.
Short explanation of the regex because you asked for one of Larkin's above:
It does exactly what you asked for - optional (K) (as (\(K\))?) followed by optional (I), etc etc. They're all captured, and they'll just be null if they're not used to match, so you can just concat them all together at the end and get your string. |
|
|
 |
charneus Wizard
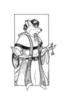
Joined: 19 Jun 2005 Posts: 1876 Location: California
|
Posted: Mon May 05, 2008 8:16 pm |
LOL. I'll try all the suggestions. Greatly appreciate this. I'm just looking for an easier way instead of creating the database and subbing the value out. :P
Charneus |
|
|
 |
gamma_ray Magician
Joined: 17 Apr 2005 Posts: 496
|
Posted: Tue May 06, 2008 4:57 am |
Fang: It'll also match absolutely nothing at all which is even more problematic than it sounds (trust me, I've had some personal experience with exactly that).
Just IMO, what you really need is some other text to match off of to tell you went to start looking, and then tack Fang's pattern on to the end. |
|
|
 |
charneus Wizard
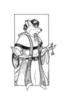
Joined: 19 Jun 2005 Posts: 1876 Location: California
|
Posted: Tue May 06, 2008 6:15 am |
Fang, tried your example, and it did not work. Vijilante's worked the best, so I'll probably continue to work with this one. Now I just need to figure out how to add color to specific characters using this trigger (if that's even possible). :P
Charneus |
|
|
 |
Fang Xianfu GURU
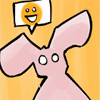
Joined: 26 Jan 2004 Posts: 5155 Location: United Kingdom
|
Posted: Tue May 06, 2008 11:34 am |
There's a bug in 2.18 that's stopping the regex from being passed to the engine properly. It's quoting the slashes for some reason. It's fixed in 2.23.
|
|
|
 |
Larkin Wizard

Joined: 25 Mar 2003 Posts: 1113 Location: USA
|
Posted: Tue May 06, 2008 11:50 am |
gamma_ray does have a point about matching on nothing, though, right?
And, I get credit for the pattern! (I only suggested %subregex because that's what was used originally. Too tired to think on my own lately, it seems.) |
|
|
 |
Fang Xianfu GURU
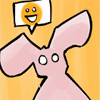
Joined: 26 Jan 2004 Posts: 5155 Location: United Kingdom
|
Posted: Tue May 06, 2008 11:55 am |
Yeah, but I assumed it'd be used as part of a larger pattern (since there has to actually be something to have the flags, and it's possible for something to have no flags). You could add a lookbehind at the end to make sure at least one of them's matched if you want to use it on its own.
|
|
|
 |
charneus Wizard
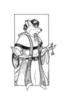
Joined: 19 Jun 2005 Posts: 1876 Location: California
|
Posted: Tue May 06, 2008 3:48 pm |
Yeah, you do get credit, Larkin. I meant to put your name in there, too. But I think I was just as tired as you are. :P
Fang, the problem with adding the larger part of the pattern is it would only be * anyway. There's no real way to add the pattern. Sure, when I look in my inventory, I can just turn the trigger on, then back off. However, I also want this for when I walk into a room. So that makes it a bit harder to match a larger pattern, hence why I only wanted to match the flags. Otherwise, it'll just be analyzing every line to see if it had flags or not.
Charneus |
|
|
 |
Fang Xianfu GURU
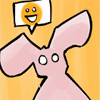
Joined: 26 Jan 2004 Posts: 5155 Location: United Kingdom
|
Posted: Tue May 06, 2008 5:18 pm |
Ah, in that case, one of the alternatives might be better. The lookbehind would mar the prettiness of mine and would slow it down.
|
|
|
 |
|
|