 |
Fang Xianfu GURU
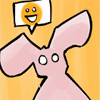
Joined: 26 Jan 2004 Posts: 5155 Location: United Kingdom
|
Posted: Sun May 27, 2007 4:20 pm
Getting Lua to work in CMUD (or zMUD!) |
Since there was some interest in getting Lua into CMUD, but the official response was a bit murky, I thought I'd look into alternatives. I thought getting this to work with any MUD would be taxing, but I really struck gold here - not only will this program work with any MUD, it'll also work with any client! So you can use this to get Lua in CMUD and zMUD! And so, without further ado, I present: MudBot! The greatest proxy the world has ever seen!
Table of Contents
1) Documentation/Sample code
2) FAQ
What is MudBot?
MudBot is a proxy - that means that you run it on your computer as well as your client. Your client connects to MudBot and MudBot connects to the server. MudBot is modular - anyone can write modules for it to do different things. The module I'm including in this version is a module that adds Lua support - when MudBot recieves lines from the server or commands from the client, it can execute whatever Lua code you write. MudBot supports MXP, MCCP and ATCP.
Download Links
MudBot is covered by the GNU GPL - as such, I have to provide source code with the binaries. Here they are:
MudBot - Probably want you want.
MudBot source.
You may want to just jump in, and that's fine. I recommend reading the documentation and the FAQ when you get the time, though.
Installation Instructions
1) Download mb.zip from the link provided above.
2) Extract it somewhere - anywhere will do. You might want to make a shortcut to mb-core.exe
3) Open config.txt. You'll see a place where you can enter your MUD's address and port - enter those and save the file.
4) Run mb-core.exe - the console should report that it's successfully entered the main loop.
5) Open zMUD or CMUD. Create a new session. The address should be "localhost" and the port "123".
6) Connect to the session you just created. MudBot should send a preamble and then you should see the standard login message from your MUD. To get help, turn off parsing and type `help. To get help for ILua, type `il help.
So how do I get Lua working?
You'll see config.ilua.txt in your MudBot directory. Open this file. All you need to do is add something like this:
Code: |
module "Test" ./test_scripts
load "test1.lua"
load "test2.lua" |
to load the script files test1.lua and test2.lua in the test_scripts folder, which is inside the MudBot folder. Modules in ILua are completely independent - they have separate global tables and any changes you make to one will not affect the other in any way.
For a more thorough explanation of how to actually make this Lua code actually do things, continue on to the documentation/sample code section. |
|
Last edited by Fang Xianfu on Tue May 29, 2007 3:40 pm; edited 4 times in total |
|
|
 |
Fang Xianfu GURU
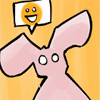
Joined: 26 Jan 2004 Posts: 5155 Location: United Kingdom
|
Posted: Sun May 27, 2007 4:21 pm |
Documentation
The original documentation for MudBot and ILua can be found on MudBot's SourceForge page.
The following is a full list of functions and tables created by ILua taken from the ILua source code, with a few explanatory additions.
version = "M.m"
Callbacks
unload = nil, - This function is executed when MudBot unloads the module for whatever reason.
server_line = nil, - This function is executed whenever a normal line is recieved from the server.
server_prompt = nil, - This function is executed whenever a prompt is recieved - that is, a line without normal end-of-line characters.
client_aliases = nil, - This function is executed whever the client sends a command. If the function returns true, don't send the command to the server.
Communication
These are pretty self-explanatory, I think. Experiment!
echo
echop
send
debug
prefix
suffix
insert
replace
show_prompt
Utility
cmp
C - This table contains colour codes. C.r is dark red, C.R is bright red, and so on. C.x is the 0 colour code - make sure you append it to anything you've used another C code in to stop the colour bleeding onto other lines
atcp - This table contains ATCP values. See the FAQ for more information on ATCP.
MXP / not implemented
Timers
add_timer - This function creates alarms. The time in seconds (can be part or whole. 3.0 is valid, as is 1.3) is the first parameter - then the function to execute when the timer finishes, and then an optional id for use with del_timer. All timers are one-shot.
del_timer
Networking / not implemented
LINE structure
line - Contains the last line received from the server. DO NOT EDIT THIS! Use the Communication functions above.
raw_line - Contains the line recieved, but with the ANSI colour codes left in.
ending - The characters that ended the line (\n etc). These aren't included in the line variable.
len - Length of line.
raw_len - Length of raw_line.
gag_line - if true, gag the line
gag_ending - if true, gag the line's ending characters
Sample Code
Triggers
You may notice that the word "trigger" hasn't been used once during the documentation above. That's because MudBot doesn't include any concept of triggers - you need to write your own trigger parser! The mb.server_line function is called whenever a line is recieved, but it takes no parameters. The mb.line variable contains the line instead - your function just needs to include some comparison against that variable. Here's a quick example that avoids the horrible if...elseif...elseif... syntax that the wiki gives as its example.
(Note: This example uses the PCRE library to create regexes for its matches. For more information on using PCRE in ILua, see the FAQ.)
Code: |
-- rex table, key = id, val = compiled rex.
trig_rex={}
-- trigger command table, key = id, val = func
trig_func={}
-- trigger event handler
mb.server_line = function ()
for id in pairs(trig_rex) do
local match
_, _, match = trig_rex[id]:match(mb.line)
if match then
trig_func[id](match)
end
end
end |
The mb.server_prompt function works in exactly the same way as mb.server_line but is only executed on prompts.
Aliases
Aliases are mentioned in the documentation, thankfully, and they work much like triggers in that the function is called whenever the a command is recieved from the client. Unlike the mb.server_line function, mb.client_aliases is given a parameter - the entire line that was sent from the client. Here's a simple alias parser:
Code: |
-- alias table. Keys are command words (first word of input), values are functions that take an array of parameters.
-- The array of parameters has two special elements. -1 is entire input line that matched the alias. 0 is the alias' command word.
alias = {}
function mb.client_aliases (input)
local i, params = 0, {}
for match in string.gmatch(input,"%S+")
params[i]=match
i=i+1
end
if alias[params[0]] then
params[-1] = input
alias[params[0]](params)
return true
else
return false
end
end |
If your mb.client_aliases function returns true, the line isn't sent to the server, just like zMUD. If you don't return true, the command is still sent to the server - useful for doing "silent alias" functions.
A test alias
Here's an alias that'll just execute whatever its parameters are. Quite useful for quick and dirty testing without having to open a text editor.
Code: |
function alias._test_exec (params)
local _, _, line = string.find(params[-1],"%s+(.+)$")
if line then
assert(loadstring(line))()
end
end |
|
|
Last edited by Fang Xianfu on Thu May 31, 2007 9:25 pm; edited 3 times in total |
|
|
 |
Fang Xianfu GURU
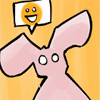
Joined: 26 Jan 2004 Posts: 5155 Location: United Kingdom
|
Posted: Sun May 27, 2007 4:22 pm |
FAQ
1) Did you create MudBot?
I most certainly did not. MudBot was originally written by Andrei Vasiliu for the MUD Imperian. It has since been modified by many people - check it out on SourceForge for more info.
2) Did you create ILua?
Another no. That honour goes to Tobiass Josten - check out the ILua page of the MudBot wiki. The version I've included here also has some edits by our own Larkin.
3) Are there any other .dll modules available for MudBot?
There are indeed. There're a number of Imperian-specific modules in the IMTS SourceForge download including a mapper and a voting system. There's also a version of the mapper adapted for the MUD Lusternia available on SourceForge.
4) I've made some changes to my scripts since I ran MudBot. Do I have to restart it to load the changes?
Absolutely not! Just turn off parsing and do `il reload module. ILua will unload the module and then reload the new files, without dropping you from the MUD!
5) How do I use PCRE with ILua?
It's easier than you think! First, download lrexlib from LuaForge. Once you've got that, copy rex_pcre.dll and pcre.dll from the zip file into your MudBot folder.
Unfortunately, rex_pcre.dll expects a different name for the lua dll - so make a copy of it and rename it to lua5.1.dll.
Now all you need to do is have one of your lua scripts execute this code:
Code: |
rex = require("rex_pcre") |
And now all the PCRE functions are loaded into the rex table. The best documentation I've found for those functions is on the MUSHClient website, here (NOTE: Both this documentation and my example above use lrexlib 1.19. Documentation for 2.1 is available on the lrexlib website, here). Since the expressions are compiled when they're created, rather than when they're run, this has the potential to be amazingly quick.
6) What's this ATCP stuff?
ATCP is an MXP-like protocol used by Iron Realms Entertainment. It sends variables to the client and so on. Since MudBot was first written for the Iron Realms MUD Imperian, it includes support for ATCP. I've turned it off in the version of MudBot available from my site - if you play an IRE MUD, you might want to turn it back on. To do so, just change the atcp_login_as line in config.txt from "none" to "default".
The version of i_lua.dll that I've included in this download is set up for Lusternia, but it should work on the other IRE MUDs too. If it doesn't, you can do a find for "atcp" in the source for ILua and edit what variables it's expecting to recieve from the server and setting for ILua to access.
7) How does MudBot tell which lines are prompts and which are normal lines?
By the characters that end the line. MUDs normally end their lines with CR or LF or both. That's one of the reasons that the ending is stored separately.
8) No matter what I do, ILua just won't run my code!
First, check if your module is loaded by turning off parsing and typing `il mods. If your module isn't listed, check that you've pointed the module line in config.ilua.txt to the right folder.
If your module's listed but your code still isn't running, you've encountered one of the few bugs in ILua. To fix it, add a few newlines to the end of config.ilua.txt and then write a comment at the bottom like "#test" and it should start working.
9) Is MudBot available for other OSes?
It is, but I have no idea if i_lua.dll is compatible with those other OSes. You'll have to do your own experimentation. |
|
Last edited by Fang Xianfu on Tue May 29, 2007 6:00 pm; edited 3 times in total |
|
|
 |
MattLofton GURU
Joined: 23 Dec 2000 Posts: 4834 Location: USA
|
Posted: Sun May 27, 2007 6:23 pm |
Quote: |
6) Connect to the session you just created. MudBot should send a preamble and then you should see the standard login message from your MUD. To get help, turn off the smart command line and type `help. To get help for ILua, type `il help.
|
So MudBot's basically a proxy? Wonder if that would work properly with Simutronics games or if we'd have to revert to the pre-Simutronics support stuff? |
|
_________________ EDIT: I didn't like my old signature |
|
|
 |
Fang Xianfu GURU
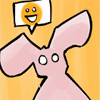
Joined: 26 Jan 2004 Posts: 5155 Location: United Kingdom
|
Posted: Sun May 27, 2007 8:19 pm |
It's a proxy, yes. I have no idea if it'd work with Simutronics - try it and see!
|
|
|
 |
Daagar Magician
Joined: 25 Oct 2000 Posts: 461 Location: USA
|
Posted: Mon May 28, 2007 2:50 pm |
I don't believe there is any reason why it wouldn't work. The only big catch is that it won't have any support for any special protocol offered by Simutronics (ie., if they have an MXP-like system, which I thought they did). However, you can choose to let that part fall-through and still let zMud/cMud handle it, or write your own handlers in Lua.
And this is true for the whole system in general... you can pawn off what you want to Lua via MudBot, but for things MudBot can't do (mapper control, etc) you still have the full power of cmud/zmud. MudBot will catch all the mud output first, do any processing you've created in Lua, then pass it to zmud/cmud where you can do any additional processing. |
|
|
 |
Fang Xianfu GURU
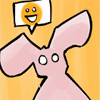
Joined: 26 Jan 2004 Posts: 5155 Location: United Kingdom
|
Posted: Mon May 28, 2007 4:47 pm |
It may be that MudBot will strip the IAC WHATEVER codes when it parses the output from the server, though, even if it doesn't understand them. I haven't tried it on muds with their own MXP-like protocols.
One of the really nice things about MudBot is that, to the client, it looks like the server is sending the info. So you can manually send the [ n z codes that MXP expects to open up the MXP functions in the client, and then use the restricted MXP tags like <!ELEMENT> from within your own scripts. I'm currently working on a way to have ILua parse rooms itself so that it can get certain information on the room, but mark them with MXP so that the mapper will pick them up with no extra configuration. |
|
|
 |
Rorso Wizard
Joined: 14 Oct 2000 Posts: 1368
|
Posted: Tue May 29, 2007 1:42 pm |
I have made a *very* experimental zLua.dll-file that integrates itself with cMUD, and let you use Lua. If you want to test it click here. As I am a super newbie to COM-programming it most likely has all kind of weird bugs and issues. As usual you use it at your own risk.
So far only one cMUD function is accessible, called "echo". It'll just echo text to cMUD's output window.
E.g echo("text string here") |
|
|
 |
Daagar Magician
Joined: 25 Oct 2000 Posts: 461 Location: USA
|
Posted: Tue May 29, 2007 1:48 pm |
Silly question... I turned off the smart command line, but am still unable to get the `il commands to work. I have to disable parsing completely... what obvious thing am I missing?
One other plea... I have ILua working fine _except_ the the PCRE lib. I have the 'require' statement, and the regex appears to be compiling, but when I try to use 're:match(string)', I get an error (paraphrasing) about 'attempt to call method match (a nil value)'. I'm completely stumped as to why it is unable to call the match method appropriately. All .dlls are present in the mudbot dir. I stripped down the code above to a very basic regex compiled statement, and a call to 'match' without luck... |
|
|
 |
Fang Xianfu GURU
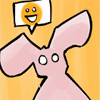
Joined: 26 Jan 2004 Posts: 5155 Location: United Kingdom
|
Posted: Tue May 29, 2007 3:38 pm |
You're not - I just assumed that turning off the smart command line would do it, and it obviously doesn't. I'll amend what it says above. You should also be able to just double the ` character to get it to work without turning off parsing.
lrexlib is a strange animal. Compiled regular expressions are stored as objects - that is, they're a value like a string or a table, and so they need to be stored somewhere. You need to do this:
someregex = rex.new("(\w+)")
Now the variable someregex contains a regular expression object. This object is what has the :match and :gmatch functions and so on, and it's that object that you need to perform the match on. So to test the line for any word, you do:
someregex:match(mb.line)
and to test for ALL words on the line, you do
someregex:gmatch(mb.line)
My example script above creates a table to store regex objects called trig_rex. trig_rex.enemyspotter = a regex object for the "enemyspotter" trigger. The script then dynamically loops through all your triggers using for in pairs() and tests all your triggers by doing trig_rex[id]:match(line) to match the line against each specific regex object. |
|
|
 |
Daagar Magician
Joined: 25 Oct 2000 Posts: 461 Location: USA
|
Posted: Tue May 29, 2007 4:23 pm |
Yep... that's exactly what I have, but it fails on somregex:match(mb.line) with the error I mentioned. I'll monkey with it some more tonight, or I may simply revert to Lua's built in pattern matching if all else fails - apparently the built in stuff is quite fast/efficient, and can do most if not all of what can be done with PCRE (just a slightly different syntax). I thought maybe I was hitting a scope error, but in Lua everything is global until you dictate otherwise so that wasn't it :) Being a newbie to Lua I'm sure isn't helping, but I certainly see the power.
Ohhh, and I just saw Rorso's reply... that is very exciting as well. |
|
|
 |
Fang Xianfu GURU
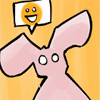
Joined: 26 Jan 2004 Posts: 5155 Location: United Kingdom
|
Posted: Tue May 29, 2007 5:58 pm |
I've found what the problem is! The latest version of lrexlib has changed some of the names of functions and removed others. I'll update the link above. Here's the pertinent part from the lrexlib manual:
lrexlib manual wrote: |
# Method match renamed to tfind
# Method gmatch removed (similar functionality is provided by function gmatch)
# Method exec: the returned table may additionally contain named subpatterns (PCRE only) |
If you want the old functionality back, use lrexlib 1.19 or earlier. The source available to download from the lrexlib site, same as 2.1. |
|
|
 |
Zugg MASTER
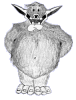
Joined: 25 Sep 2000 Posts: 23379 Location: Colorado, USA
|
Posted: Tue May 29, 2007 7:14 pm |
Fang, this was a really useful post and has given me more ideas for Lua (and the other scripting language interface with CMUD).
Just wanted to make a couple comments about this though:
Don't get too carried away trying to use MUDBot for "triggers". Remember that by intercepting the raw data from the MUD and processing it within MUDBot, you are essentially calling a scripting system when each line is received from the MUD. It is much more efficient to just use triggers within CMUD itself. CMUD already uses PCRE (it is compiled within CMUD so there is no DLL needed). And because CMUD is compiled (in Delphi...not talking about compiled scripts here), the testing of your regular expression triggers will always be faster than what Lua or MudBot can perform.
If there is a reason why you think it's better to use MudBot for triggers, then let me know (maybe start another thread). But I can't really think of any reason why you'd want to do this. It's just going to make your Mud session slower, and make it harder to share your scripts with other players.
Anyway, I'll be watching this thread to see what people end of doing with this. I'm always interested to see why something like this is desired when you can already do most of it in CMUD/zMUD already.
When using PCRE, CMUD also has "compiled" your regular expressions. This is also true for normal CMUD triggers (which are internally converted to compiled PCRE expressions). In fact, even zMUD uses compiled PCRE. |
|
|
 |
Daagar Magician
Joined: 25 Oct 2000 Posts: 461 Location: USA
|
Posted: Tue May 29, 2007 8:21 pm |
To help derail this thread entirely...
Zugg, I'll give you a high-level view of why something like this _seems_ promising to (though note that I haven't actually done anything useful with it... it was more an excuse to tinker around with lua).
Basically, I like the simplicity of being able to open up a file and have all the code right there. The GUI interface of zmud/cmud is great, especially when new to the software, but after I while I want to be able to open up "myMudStuff.script" and just write the code there. Note that I just got myself over to cmud (finally), so maybe this is possible now in cmud and I'm just behind the times (very likely - feel free to tell me to RTFM). I know Larkin for example distributes all his scripts in a few files but I'm not sure if he actually is able to _code_ them in that manner. In short - I want to pop up a developer-like IDE and just write. For example, creating a multi-step trigger in code:
#regex {whatever}...
#cond {whatever}...
#cond {whatever}...
is much quicker than going through the GUI to do the same. I did notice you have what is essentially SciTE available as an editor, so what I desire might actually be there... I've been away too long!
I can't comment on performance... I've never written anything so huge as to hit any major issues, nor played in an environment where fastest code wins. However, the IRE mud folks (achaea/lusternia/imperian) do have monster(!) scripts and there are claims of slowdowns/etc once certain magic numbers are reached that aren't present in other clients. This may be a case of perception over reality of course, and I don't believe I've seen anything with hard numbers.
As for the PCRE discussion in this thread, I don't think it was meant in any way in comparison to how zmud/cmud does things. In this case, it was a matter of "here is how you get PCRE working in Lua", as Lua out-of-the-box uses something close to, but not quite exactly unlike PCRE. Since zmud/cmud does use PCRE, lots of folks are already more familiar with that syntax and hence would want it available in Lua as well. I muddied the waters by pointing out my inability to read the lregexp docs myself. ;) |
|
|
 |
Fang Xianfu GURU
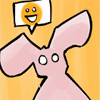
Joined: 26 Jan 2004 Posts: 5155 Location: United Kingdom
|
Posted: Tue May 29, 2007 9:18 pm |
Part of the appeal of MudBot is that it's available and working right now. It provides a stop-gap for people to be able to use Lua while they're waiting for it to be available in CMUD. That was the reason I went on the search for something like this in the first place.
To answer Daagar's question about Larkin's scripts - they're just exported textfiles from zMUD (except made to work right :P) and equivalent files hand-written for CMUD. They're just a big list of #trig commands and so on. I definitely agree about the appeal of script files. MUSHClient already incorporates an element of this, allowing you to load a script file (though I'd prefer if the client itself could load more than one file, to save using require()) and then set each function or trigger to activate a particular function. I'd much prefer that method of Lua scripting. While it wouldn't be integral like the .pkg format intends to be, I already distribute my scripts as .zip files since I make things like sound packs and icon packs available with them. Adding some text files doesn't really put me out any.
The main draw of Lua versus zScript is how easy it is to make complex data structures in Lua. I like my scripts to use as few global names as possible and Lua makes that a doddle. Metatables also add extra flexibility if you need it.
And yes, I didn't mean to compare ILua to CMUD for speed. If I'm perfectly honest, those players who've hit those magic numbers are doing something very wrong with the way they set out their triggers. You don't need that many. |
|
|
 |
Larkin Wizard

Joined: 25 Mar 2003 Posts: 1113 Location: USA
|
Posted: Wed May 30, 2007 11:19 am |
None of my scripts for zMUD/CMUD are exported text files, but close enough. I hand code everything in SciTE, outside of the clients, and import the scripts when I'm done editing.
My attraction to the ILua module has been the ability to intercept ATCP (Iron Realms-specific telnet codes) and replace my prompt with a far superior prompt. The prompt replacement is something done much easier in ILua than zMUD/CMUD. For regular triggers, aliases, and such, I would definitely still use the client and not ILua.
Still looking forward to Lua in CMUD! *hold breath* |
|
|
 |
Rorso Wizard
Joined: 14 Oct 2000 Posts: 1368
|
Posted: Wed May 30, 2007 12:07 pm |
Larkin wrote: |
Still looking forward to Lua in CMUD! *hold breath* |
I made an experimental Lua implementation(http://www.silverbridge.org/~varmel/zmud/zLua.zip) that adds itself to the script combobox and runs within cMUD. It now imports the methods in the Sess namespace so stuff like EchoStr("test", 1) should work. |
|
|
 |
Daagar Magician
Joined: 25 Oct 2000 Posts: 461 Location: USA
|
Posted: Wed May 30, 2007 2:47 pm |
Larkin, thanks for the insight.
CMUD does have some nice editor improvements over zMud (now that I'm using it a bit more), so it isn't as dramatic as my earlier post may have made it seem. However, I would love if there was a way to use the SciTE-like editor that is included with cmud to work against the scripts directly (ie., add buttons/command to automatically import/export scripts to/from cmud). The advatage of this over Larkin's method would be the automatic syntax checking and zScript highlighting and of course the fact that it is a nice all-in-one solution. This may have been the intent all along and I'm just jumping the gun... |
|
|
 |
Fang Xianfu GURU
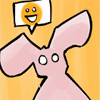
Joined: 26 Jan 2004 Posts: 5155 Location: United Kingdom
|
Posted: Wed May 30, 2007 4:12 pm |
Well, I suppose it's possible that Larkin's written a zScript.properties file for SciTE so that it syntax highlights and folds the code and things properly. That'd be seriously neat.
I don't know why I'm so hung up on having things in text files. In many ways, having your scripts represented by a GUI in CMUD is much more convenient. I guess it's because CMUD's way for making a snippet of code where you'd create a function in most languages is to create an alias (or occasionally a function). I don't like the idea of having all those aliases hanging around that you're never actually going to call directly. I remember someone saying that they used events for non-call aliases, but I don't think that's much of an improvement and just as cluttered. I like being able to shove all the functions I'm using into tables so they don't hang around polluting the namespace.
And can you believe I'd forgotten all about ATCP? That's one of the best parts about MudBot :) You could potentially add support for any custom protocol to MudBot, whereas CMUD's negotation isn't accessible (I was wondering if a plugin could do it, but apparently not) so support for this has to be added by the developer. Kinda defies the point of adding MXP support to the client really :P |
|
|
 |
shalimar GURU
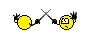
Joined: 04 Aug 2002 Posts: 4691 Location: Pensacola, FL, USA
|
Posted: Wed May 30, 2007 6:41 pm |
Couldnt you just make said function off of a local variable?
|
|
_________________ Discord: Shalimarwildcat |
|
|
 |
Larkin Wizard

Joined: 25 Mar 2003 Posts: 1113 Location: USA
|
Posted: Thu May 31, 2007 2:24 am |
Anonymous functions (sometimes called lambda functions or delegates) are very cool and one of the reasons I enjoy coding in Lua.
|
|
|
 |
Zugg MASTER
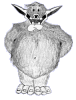
Joined: 25 Sep 2000 Posts: 23379 Location: Colorado, USA
|
Posted: Thu May 31, 2007 4:58 pm |
Can anyone send me a link to more info on ATCP? I don't know anything about this, and if it's something useful to add to CMUD then I should learn more about it.
Edited: BortaS sent me the ATCP link. Looks trivial to implement as another type of trigger in CMUD.
Thanks for this discussion...it has been very interesting for me. But I'm going to go start another thread about some of this so that this thread can continue discussion of Fang's LUA/MudBot stuff. Sorry for hijacking the thread. |
|
|
 |
Fang Xianfu GURU
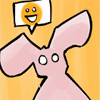
Joined: 26 Jan 2004 Posts: 5155 Location: United Kingdom
|
Posted: Thu May 31, 2007 6:46 pm |
Assuming that this link was the one that BortaS sent you, I don't think that tells the entire story. MudBot has an atcp_login_as variable, so presumably the server is expecting some kind of ATCP-based response to validate ATCP support. Here's a snippet of the MudBot code that seems to be building a response to an auth request:
Code: |
char sb_atcp[] = { IAC, SB, ATCP, 0 };
sprintf( buf, "%s" "auth %d %s" "%s",
sb_atcp, atcp_authfunc( body ),
atcp_login_as, iac_se );
send_to_server( buf ); |
and here's atcp_authfunc, just for completeness
Code: |
int atcp_authfunc( char *seed )
{
int a = 17;
int len = strlen( seed );
int i;
for ( i = 0; i < len; i++ )
{
int n = seed[i] - 96;
if ( i % 2 == 0 )
a += n * ( i | 0xd );
else
a -= n * ( i | 0xb );
}
return a;
} |
But I've no idea what's going on there other than that it's iterating through all the characters of the "body" that the server's sent for authentication and doing something involving modulus and bitwise OR to them. Either way it seems like IRE is taking minor pains to keep unofficial clients out, so it might be wise to ask them about it. They haven't minded people using MudBot, so I can't imagine that they'd mind zMUD supporting it either. But then zMUD is a commercial product and MudBot isn't.
EDIT: I just added a little alias that's useful for quick testing to the sample code section above. |
|
|
 |
Zugg MASTER
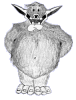
Joined: 25 Sep 2000 Posts: 23379 Location: Colorado, USA
|
Posted: Fri Jun 01, 2007 5:23 pm |
Just to finish the side-track on ATCP: I contacted the owner of IronRealms and have gotten the official go-ahead to put ATCP support into CMUD. I should be receiving the technical details from the Achaea manager soon. I'm sure all of the Achaea players will be happy to hear this.
When I implement this, I also plan to add more generic support for responding to other telnet option negotiation within CMUD. |
|
|
 |
Daagar Magician
Joined: 25 Oct 2000 Posts: 461 Location: USA
|
Posted: Fri Jun 01, 2007 7:36 pm |
Wow, that's excellent news on both your and IRE's part. I know there are other ATCP-like systems out there that have been made proprietary purposely to _block_ clients like cmud from using them :(
|
|
|
 |
|
|
|
You cannot post new topics in this forum You cannot reply to topics in this forum You cannot edit your posts in this forum You cannot delete your posts in this forum You cannot vote in polls in this forum
|
|