 |
Salaric Beginner
Joined: 10 Jun 2008 Posts: 17
|
Posted: Tue Jun 10, 2008 10:02 pm
Script help - math confusion |
I'm trying to make a script that will scan my Achaean rift for the necessary ingredients and tell me how many health/mana/etc refills I can make. I've made something that works semi-decent with health and mana, but I have to manually change the trigger to whatever ingredient I have the least of. I'm horrid with math and I'm trying to figure out how to make zmud scan the four ingredients, figure out which one has the least then divide that by five. This is what I have so far:
Rift looks like this:
Code: |
[1533] bayberry bark [2000] bellwort flower [1244] black cohosh
[1998] bloodroot leaf [ 8] blue ink [ 9] cloth
[ 1] crystal pentagon [1999] echinacea [2000] ginger root
[1490] ginseng root [1998] goldenseal root [ 1] green ink
[1995] hawthorn berry [ 505] irid moss [ 407] kuzu root
[2000] lady's slipper [ 267] lobelia seed [1994] myrrh gum
[1909] prickly ash bark [ 7] red ink [ 1] rope
[ 64] skullcap [ 552] valerian [ 19] venom sac
[ 3] yellow ink |
#CLASS {Fills}
#VAR health {0}
#VAR mana {0}
#ALIAS fills {IR;#ECHO ;#ECHO You can make @health health superfills;#ECHO You can make @mana mana superfills;health=0;mana=0}
#TRIGGER {(%d)~]valerian} {#AD health (%1 / 5)}
#TRIGGER {(%d)~] bloodroot} {#AD mana (%1 / 5)}
#CLASS 0
It's good enough to work, though trying to make it better. Also, here's another math part I'm having issues trying to figure out. Epidermal requires 2 kuzu roots; 1 bloodroot leaf; 1 hawthorn berry; 1 ginseng root. I tried to use this to make it first divide how many kuzu I had by 2, then divide that number by five. But it didn't seem to catch the second division.
#TRIGGER {(%d)~]kuzu root} {#AD epidermal (%1 / 2 / 5 )}
Any thoughts on how I can make it scan my rift so I didn't have to manually change the trigger line and/or how to do the extended math like the kuzu above? I figure that I need to make it load the four numbers in to four seperate variables and then do the math with that, just not sure how.. Any help would be appreciated |
|
|
 |
Fang Xianfu GURU
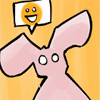
Joined: 26 Jan 2004 Posts: 5155 Location: United Kingdom
|
Posted: Tue Jun 10, 2008 11:03 pm |
In future, please use the code tag when spacing is important. I've added one for you for now.
The simplest way is to work out something that automatically fills up a variable with the values of everything in your rift. Once you've got that, you can use that variable to query what you have in your rift at any given time. So, here's a trigger that'll do just that:
Code: |
#regex {^ \[\s*(\d+)\] ((?:\w+ )+)\s*(?:\[\s*(\d+)\] ((?:\w+ )+)\s*)?(?:\[\s*(\d+)\] ((?:\w+ )+)\s*)?} {#addkey riftstuff {%2} {%1};#if (%4) {#addkey riftstuff {%trim(%4)} {%3}};#if (%6) {#addkey riftstuff {%trim(%6)} {%5}}} |
You'll want to enable and disable this trigger as appropriate so that it doesn't trigger when you're not displaying your rift - though it probably won't anyway, since most lines don't start with two spaces.
Anyway. Now you've got a variable to check, you just check it. For example:
#say You have %db(@riftstuff,"bayberry bark") bayberry and can make %eval(%db(@riftstuff,"bayberry bark")/4) of some elixir that requires four bayberry. |
|
|
 |
Fang Xianfu GURU
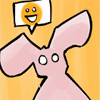
Joined: 26 Jan 2004 Posts: 5155 Location: United Kingdom
|
Posted: Tue Jun 10, 2008 11:09 pm |
Hmm... seems to be a problem with that regex that stops it from working properly if all three items aren't suppied. Try this one instead:
Code: |
^ \[\s*(\d+)\] ([\w' ]+)\s*(?:\[\s*(\d+)\] ([\w' ]+)\s*)?(?:\[\s*(\d+)\] ([\w' ]+)\s*)? |
another small change, because I realised that some of your words contain apostrophes. |
|
|
 |
Salaric Beginner
Joined: 10 Jun 2008 Posts: 17
|
Posted: Tue Jun 10, 2008 11:28 pm |
Wow! Thank you, I believe this helps a lot
|
|
|
 |
Salaric Beginner
Joined: 10 Jun 2008 Posts: 17
|
Posted: Wed Jun 11, 2008 9:36 pm |
One more thing, I'm trying to make a table and I've been searching around for a function or something that will allow me to space things. This is what it looks like now
Code: |
Herb -------------Rift--$$$---
---------------------------
Myrrh -------------1949----10---
Echinacea ---------1956----05---
Ginseng -----------1863----35---
Hawthorn ----------1990----05---
Bayberry ----------1720----05---
Elm ---------------15----15---
Lobelia -----------488----10---
Kelp --------------20----45---
Weed --------------1----10---
Pear --------------95----15---
Goldenseal --------1925----35---
Sileris -----------40----30---
Ash ---------------1985----15---
Cohosh ------------1508----15---
Skullcap ----------309----20---
Bellwort ----------1995----20---
Bloodroot ---------1991----30---
Moss --------------714----45---35700gs
Valerian ----------610----30---
Kola --------------30----20---
|
I'm trying to make it look like this, and I know I can't just use the spacebar:
Code: |
Herb Rift Per $$$
------------------------------------
Myrrh 1949 10 3000gs
Echinacea 1956 05 3000gs
Ginseng 1863 35 3000gs
Hawthorn 1990 05 3000gs
Bayberry 1720 05 3000gs
|
Anyone know of the fuction I need to use to do this spacing?[/quote] |
|
|
 |
Salaric Beginner
Joined: 10 Jun 2008 Posts: 17
|
Posted: Wed Jun 11, 2008 9:37 pm |
Ack, the spacing didn't show up here either. In any case, is there a function for spacing in #ECHO?
|
|
|
 |
Vijilante SubAdmin

Joined: 18 Nov 2001 Posts: 5182
|
Posted: Wed Jun 11, 2008 10:00 pm |
See the %format help. Also you can use the %min function to determine which is the lowest value. Working off of Fang's trigger the code would look something like
Code: |
#ALIAS CanBrew {
#VAR TempPot {}
#LOOPDB @RiftStuff {
#IF (%db(@{%1},%key)) {
#ADDITEM TempPot {%eval(%val/%db(@{%1},%key))}
}
#IF (@TempPot) {
#SHOW {You have ingredients to brew %min(%replace(@TempPot,"|",",")) of %1}
} {
#SHOW {Potion %1 was unknown.}
} |
|
|
_________________ The only good questions are the ones we have never answered before.
Search the Forums |
|
|
 |
Fang Xianfu GURU
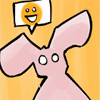
Joined: 26 Jan 2004 Posts: 5155 Location: United Kingdom
|
Posted: Thu Jun 12, 2008 12:01 am |
It's code, not quote - but thanks for making the effort ;)
Yeah, %format's pretty easy to use. %format("&80s",string) will give you right alignment, and &-80s will give you left alignment with a bunch of trailing spaces. Centre alightment is more tricky - you essentially want to use right-alignment, but you want to make sure that the middle of the string lines up with the 40th character. So you take the length of the string, divide by 2, add that to 40, and that's your value for "&nns". You'll not be able to use this if your strings are longer than 80 characters, but they won't be so that's fine. The code for that will look something like:
#say %format(%concat("&",%eval(40+(%length(%-1)/2)),"s"),%-1)
Another method I've seen people use, which you might prefer, is to use %repeat. So:
#say %concat(%repeat(" ",(80-%length(string))),string)
but it's quite ugly and just does the same thing as %format. |
|
|
 |
Salaric Beginner
Joined: 10 Jun 2008 Posts: 17
|
Posted: Fri Jul 02, 2010 4:51 pm |
I've recently had to switch from zmud to cmud when I installed windows 7 and the REGEX I was given here doesn't seem to work quite right anymore. It adds extra spaces at the end of variable names. I'll use an underscore to represent the spaces after the word
hawthorn berry_____
kola nut___________
ginger root________
etc.
It doesn't do this with every word but it does with several and it's making it difficult for me to access the variable with %db. Any suggestions?
This is the code I'm using -
#REGEX {^ [\s*(\d+)] ([\w' ]+)\s*(?:[\s*(\d+)] ([\w' ]+)\s*)?(?:[\s*(\d+)] ([\w' ]+)\s*)?} {#addkey riftstuff {%2} {%1};#if (%4) {#addkey riftstuff {%trim(%4)} {%3}};#if (%6) {#addkey riftstuff {%trim(%6)} {%5}}} "Merchant"
Code: |
Glancing into the Rift, you see:
[ 200] bayberry bark [ 200] bellwort flower [ 299] black cohosh
[ 199] bloodroot leaf [ 1] blue ink [ 498] echinacea
[ 700] ginger root [ 47] ginseng root [ 396] goldenseal root
[ 300] hawthorn berry [ 16] irid moss [ 98] kelp
[ 200] kola nut [ 300] kuzu root [ 499] lobelia seed
[ 498] myrrh gum [ 500] prickly ash bark [ 197] prickly pear
[ 1] red ink [ 100] sileris [ 298] skullcap
[ 500] slippery elm [ 298] valerian [ 1] yellow ink
|
|
|
|
 |
MattLofton GURU
Joined: 23 Dec 2000 Posts: 4834 Location: USA
|
Posted: Fri Jul 02, 2010 5:22 pm |
use the %trim() function.
|
|
_________________ EDIT: I didn't like my old signature |
|
|
 |
Salaric Beginner
Joined: 10 Jun 2008 Posts: 17
|
Posted: Fri Jul 02, 2010 5:45 pm |
Sorry for my ignorance, but how exactly?
|
|
|
 |
MattLofton GURU
Joined: 23 Dec 2000 Posts: 4834 Location: USA
|
Posted: Fri Jul 02, 2010 5:50 pm |
%trim(%1). You use it in your trigger code, not in the pattern.
|
|
_________________ EDIT: I didn't like my old signature |
|
|
 |
Salaric Beginner
Joined: 10 Jun 2008 Posts: 17
|
Posted: Fri Jul 02, 2010 6:12 pm |
Wonderful! Thank you very much
|
|
|
 |
|
|