 |
chamenas Wizard
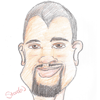
Joined: 26 Mar 2008 Posts: 1547
|
Posted: Sun Nov 02, 2008 6:46 pm
IF Statements and Commands |
Can I put an IF statement in a command like "writelog" ? The reason I ask is because I'm thinking about coloring some things in the write log as they would appear in the game. The fundamental issue with this, however, is that I sometimes have my vitals (hp, mana, movement) in my prompt for purposes of people viewing combat logs. I want to make it so that those numbers turn yellow and then red based on a percentage. Thus I have to do a bit of a division problem in the #WRITELOG statement and use #IF to choose which way to color it.
|
|
|
 |
charneus Wizard
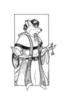
Joined: 19 Jun 2005 Posts: 1876 Location: California
|
Posted: Sun Nov 02, 2008 7:12 pm |
Use the %if function for that.
Charneus |
|
|
 |
chamenas Wizard
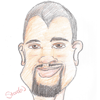
Joined: 26 Mar 2008 Posts: 1547
|
Posted: Sun Nov 02, 2008 9:26 pm |
There's going to be a ton of functions in there
It would be:
#WRITELOG @time | %if((%somedivfunction(n1,n2)>somepercent) AND (%somedivfunction(n1,n2)>somepercent), %ansi(color))%if((%somedivfunction(n1,n2)>somepercent) AND (%somedivfunction(n1,n2)>somepercent), %ansi(color))n1/n2%ansi(hi, red)hp%ansi(reset) | %if((%somedivfunction(n3,n4)>somepercent) AND (%somedivfunction(n3,n4)>somepercent), %ansi(color))%if((%somedivfunction(n3,n4)>somepercent) AND (%somedivfunction(n3,n4)>somepercent), %ansi(color))n3/n4%ansi(hi, blue)mana%ansi(reset) | %if((%somedivfunction(n5,n6)>somepercent) AND (%somedivfunction(n5,n6)>somepercent), %ansi(color))%if((%somedivfunction(n5,n6)>somepercent) AND (%somedivfunction(n5,n6)>somepercent), %ansi(color))n5/n6$ansi(gray)mv%ansi(reset)
Looks about right, yes? |
|
|
 |
charneus Wizard
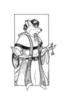
Joined: 19 Jun 2005 Posts: 1876 Location: California
|
Posted: Sun Nov 02, 2008 9:36 pm |
Looks right, but you could probably even shorten that up with a function or two. Just a thought.
Charneus |
|
|
 |
chamenas Wizard
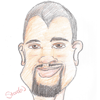
Joined: 26 Mar 2008 Posts: 1547
|
Posted: Sun Nov 02, 2008 10:07 pm |
I haven't played with functions much.
|
|
|
 |
Tarn GURU
Joined: 10 Oct 2000 Posts: 867 Location: USA
|
Posted: Mon Nov 03, 2008 1:09 am |
chamenas wrote: |
There's going to be a ton of functions in there [:P]
It would be:
#WRITELOG @time | %if((%somedivfunction(n1,n2)>somepercent) AND (%somedivfunction(n1,n2)>somepercent), %ansi(color))%if((%somedivfunction(n1,n2)>somepercent) AND (%somedivfunction(n1,n2)>somepercent), %ansi(color))n1/n2%ansi(hi, red)hp%ansi(reset) | %if((%somedivfunction(n3,n4)>somepercent) AND (%somedivfunction(n3,n4)>somepercent), %ansi(color))%if((%somedivfunction(n3,n4)>somepercent) AND (%somedivfunction(n3,n4)>somepercent), %ansi(color))n3/n4%ansi(hi, blue)mana%ansi(reset) | %if((%somedivfunction(n5,n6)>somepercent) AND (%somedivfunction(n5,n6)>somepercent), %ansi(color))%if((%somedivfunction(n5,n6)>somepercent) AND (%somedivfunction(n5,n6)>somepercent), %ansi(color))n5/n6$ansi(gray)mv%ansi(reset)
Looks about right, yes? |
If it were me, I would do that in a few steps and add a few variables which store the intermediate results.
Until you actually prove that there's a performance problem, clarity >> optimization.
-Tarn |
|
|
 |
Fang Xianfu GURU
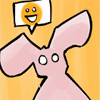
Joined: 26 Jan 2004 Posts: 5155 Location: United Kingdom
|
Posted: Mon Nov 03, 2008 1:41 am |
Mmm, you can do your calculations with commands beforehand, storing the string in a variable, then write the contents of the variable. Eg:
$string=""
#if (something) {$string=%concat($string,"Something: Yes")} {$string=%concat($string,"Something: No")}
#if (something else) {$string=%concat($string," Something Else: Yes")} {$string=%concat($string," Something Else: No")}
#writelog $string |
|
|
 |
chamenas Wizard
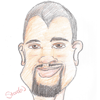
Joined: 26 Mar 2008 Posts: 1547
|
Posted: Mon Nov 03, 2008 3:20 am |
Where should I do the calculation? What's the division function in CMUD?
right now the IF statements would be
#IF ((n1/n2<=.75) AND (n1/n2>.50)) {color it yellow}
#IF (n1/n2<=.50) {color it red} |
|
|
 |
intoK Apprentice
Joined: 18 Feb 2007 Posts: 190
|
Posted: Mon Nov 03, 2008 9:12 am |
you really should reduce amount of calculations
calc percentages for hp,mn before concating
fe.
$hpp = (n1/%float(n2))
then inline switch
"__string___", %switch($hpp<0.5,%ansi(colour low),$hpp<0.75,%ansi(colour med),1,%ansi(colour default))n1~/n2,"hp " etc etc etc
you do fp division by turning one of integers to float with %float() as above |
|
|
 |
chamenas Wizard
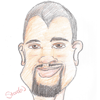
Joined: 26 Mar 2008 Posts: 1547
|
Posted: Tue Nov 04, 2008 3:33 pm |
Here's what I came up with
Code: |
#IF ((@hp_max/@hp_curr)<0.75) AND (@hp_max/@hp_curr)>=0.50))
{
$hp_string=%ansi(hi, yellow)@hp_curr%ansi(reset)/@hp_max%ansi(hi, red)hp%ansi(reset)
}
#IF ((@hp_max/@hp_curr)<0.50))
{
$hp_string=%ansi(hi, red)@hp_curr%ansi(reset)/@hp_max%ansi(hi, red)hp%ansi(reset)
}
#IF ((@mana_max/@mana_curr)<0.75) AND (@mana_max/@mana_curr)>=0.50))
{
$mana_string=%ansi(hi, yellow)@mana_curr%ansi(reset)/@mana_max%ansi(hi, blue)mana%ansi(reset)
}
#IF ((@mana_max/@mana_curr)<0.50))
{
$mana_string=%ansi(hi, red)@mana_curr%ansi(reset)/@mana_max%ansi(hi, blue)mana%ansi(reset)
}
#IF ((@mv_max/@mv_curr)<0.75) AND (@mv_max/@mv_curr)>=0.50))
{
$mv_string=%ansi(hi, yellow)@mv_curr%ansi(reset)/@mv_max%ansi(gray)mv%ansi(reset)
}
#IF ((@mv_max/@mv_curr)<0.50))
{
$mv_string=%ansi(hi, red)@mv_curr%ansi(reset)/@mv_max%ansi(gray)mv%ansi(reset)
}
#WRITELOG > @Time | $hp_string | $mana_string | mv_String
|
|
|
|
 |
|
|
|
You cannot post new topics in this forum You cannot reply to topics in this forum You cannot edit your posts in this forum You cannot delete your posts in this forum You cannot vote in polls in this forum
|
|