Zugg MASTER
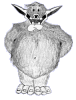
Joined: 25 Sep 2000 Posts: 23379 Location: Colorado, USA
|
Posted: Fri Oct 31, 2008 10:03 pm
Life as a web developer |
Now that the www.slightlymorbid.com site is public, I can finally talk about this whole experience. It was hard working on that site without any place to vent to ;)
So, this is the first in a series of articles that I'm going to post about web development and web design. It was a very interesting experience and I want to share some of what I learned.
Choosing a Framework
Earlier this year, I posted the article 'New web development - Ruby on Rails?' to discuss the web framework that I was looking at for the MyMuds.com project. At the time I got excited about Ruby and Rails, but towards the end I became discouraged by the performance and the infrastructure/server needs for RoR. Then the MyMuds.com project got postponed, so I didn't look into it any further.
For the SlightlyMorbid.com project I took another look at all of this. I quickly agreed that the performance issues of RoR were bad for this project, which potentially could have a large number of users. So I started looking into PHP frameworks. I looked at Symfony (as suggested in the RoR blog entry), and also CakePHP. I liked the MVC (model-view-controller) architecture of both, but I also felt that both were a bit "heavy" and included a bunch of stuff that I didn't really want or need. In addition, Symfony was only for PHP 5. And while I want to move towards PHP 5 in the future, the current Zuggsoft site doesn't work with PHP 5 (and never will until I rewrite it). Since I wanted to run the SlightlyMorbid site on the same server to start with, I needed something that would still work with PHP 4.
After much searching, I came across a real gem. It's called CodeIgniter and it a very light-weight framework for both PHP 4 and 5. It uses the MVC model, and uses a nice URL structure that gets away from query strings, creating more search-friendly URLs. It's very small and fast.
The more I started using CodeIgniter, the more I fell in love with it. I actually love the fact that it doesn't use any template engine (like Smarty). It's just all PHP. Why bother with a template when you can just write straight PHP code? That's one less new syntax to learn and support. Since Views are separate from the main application code and object models, it's easy to keep the code separate from the design. And using the short tags in PHP makes the pages look just as good as using a template engine (at least in my opinion). Plus you get the full power of PHP at the same time.
In general, the CodeIgniter framework is less strict that other frameworks. You can use the parts that you need without being burdened with stuff that you don't want. Their base code is nicely split into small libraries that are well commented and easy to override and customize without modifying their original code. You can also add your own libraries and "helper" modules. For example, I replaced their default Session library with an improved library written by someone else because I needed more secure database-based sessions for SlightlyMorbid.
Some people complain about the fact that CodeIgniter forces you to use a certain URL structure for your application. I actually liked that. But when I was implementing the PayPal interface, I ran into situations where I needed to access GET variables passed via query strings. Fortunately, it was a simple change to CI to get it to handle this correctly. So now I have a modified framework that supports both the normal URL structure, as well as a traditional query-string URL structure. Again, it was easy to understand and modify the core files to handle this.
Finding a PHP IDE
After I found the CodeIgniter framework, it was time to pick an IDE. When I was playing with Ruby on Rails, I had installed the free Aptana Studio IDE. I upgraded it and installed the PHP plugin and used that for my IDE during the entire SlightlyMorbid project. It worked well, although it's a bit sluggish sometimes because its running in Java. I also found that I had to close it and reopen it now and then because the memory footprint kept increasing. Either some minor memory leak in Aptana, or in the Java runtime itself...hard to tell. Still, I could easily run it for several hours.
The next article in this series will talk more about PHP IDEs. Since using Aptana I have tried several others and have enough comments on it to make a complete article on picking a good IDE.
The process of building SlightlyMorbid.com
Once I had the tools, it was time for the design and coding of the SlightlyMorbid.com site. The site itself isn't very complicated. It's mainly just a email contact database with a secure session and user management system. It actually only took me a week to write all of the core PHP code (and not really a full week...more like 5 days).
Then came the hard part...and the part that I severely underestimated. I wanted the site to look nice. And we all know what a "perfectionist" I am. So lots of little details started to bug me. For example, by default when you place a text label next to a form input field in HTML, they do not line up vertically. Not in any browser. And each browser has a slightly different vertical offset.
I wanted to have nice looking HTML input forms. I wanted the labels to be in the left column and right-aligned, with the form fields in the right column. I wanted the form fields, especially TextArea fields, to expand and contract with the size of the browser window. I wanted the left column with the labels to be fixed-width, but to expand/contract with changes to the font size in the browser.
It took me 2 days just to get all of the CSS for this working properly on all browsers. And this was the way the next entire week went for me...I spent all of my time tweaking CSS for browsers. And that resulted in one primary summary comment: IE6 MUST DIE!. That browser caused me more grief that I could believe. IE7 was also a problem, but not as bad. But I was still forced to create an IE-only CSS file just to handle it's quirks. And several IE bugs in tables forced me to completely change certain design elements. For example, if you have a fixed-width column in a table, and then later use COLSPAN to span multiple columns, IE resets the width of the fixed-width column, screwing up the entire table layout.
In the end I was able to get a mostly table-free CSS layout, except for the pages that actually contain tabular data. I was also able to implement some AJAX code with the checkbox grid so that the backend database would update as soon as you clicked on a checkbox, rather than having to use a Submit button for the entire grid. While the site requires JavaScript for full functionality, I was able to make the site mostly functional without it. And I focused on accessibility so the entire site can be used by anybody.
I also spent several days getting the entire site to properly "validate". The best Firefox plugins are "Firebug" for Javascript debugging and general usefulness (I can't live without Firebug anymore), and the "Html Validator" plugin. This last plugin uses the same rules as the W3C Validation site and adds an item to the status bar at the bottom of the browser to tell you what errors/warnings a site has. I've been amazed at how many errors most sites have. Not all errors are necessarily bad. For example, Google performs some optimization on their pages that cause validation errors.
But this tool helped me find several validation errors that *were* a problem. When I discovered that the simple U tag for underlining text was depreciated and causing validation errors, I almost gave up (the W3C committee must be on drugs or something to get rid of U...what's next, getting rid of B and I and all other original HTML markup? give me a break). But ultimately I decided I wanted the site to be fully validated HTML 4.0 to give me the least trouble when getting it to work on other browsers in the future, especially mobile browsers.
I'd say that overall I probably spent more than 50% of my total development time, including debugging and testing, on CSS/Browser issues. That's very sad. I expected this to be less of a problem these days with all of the browser improvements. And even though Internet Explorer was the biggest source of problems, I still even had some issues between FireFox 2.x and FireFox 3.x. They have made some changes to fonts and vertical alignment that prevent my nice HTML forms from lining up in FireFox 3. I was impressed with Google Chrome and Apple Safari. Neither of those browsers gave me trouble once I had the site working on Firefox. So that's a good sign for the future. Maybe Google can make some deals with Dell to get Chrome preinstalled and the market share for IE will eventually decrease. Or maybe we'll see a miracle and IE8 will actually be better.
It was fun!
I've worked in web development for a long time. Back at Los Alamos National Lab, I created their first web site and started what eventually became an entire group focused on web development. I've taught HTML classes and was even involved in the early web standards process for a short time. That was long ago.
In the past several years I've mostly just focused on our own internal Zuggsoft site. But I've also created web sites for non-profit SCA groups, and for other small businesses. The SlightlyMorbid.com site is the latest and most modern site I've created, and it was actually fun to get back into web design again.
While I remain committed to the CMUD project, I've renewed my interest in eventually writing the MyMuds.com site and also to start slowly improving the Zuggsoft site itself. It really needs to be rewritten from scratch someday so that I can get away from the PHPBB and MXPortal history. But that's a big project and won't happen until after MyMuds.com, which won't happen until after the CMUD mapper.
It was nice to have a change of pace. It helped refresh my brain and made working on the mapper more fun too. So you'll see more web stuff from me in the future. |
|