 |
mabort Novice
Joined: 08 Mar 2001 Posts: 44
|
Posted: Wed Apr 26, 2006 11:17 pm
Variable expansion problem. |
Alright, I'm about to pull my hair out. Let me describe this problem before I show you the code...
I'm trying to create an alias that will start an autoroller for character generation. What is needs to do is this:
(1) Take in data from the user as to what they prefer the stats to at the least be,
(2) Generate a condition statement that will evaluate whether or not the stats they want have been produced,
(3) Set this variable as the response to a trigger that is already predefined.
The first part and the third part are no problem. The problem arises from trying to do the second part. I can get it to do pretty much everything I want it to do except NOT expand a variable name that is used in the conditional evaluation. Here is the code:
Code: |
#var strExpected %prompt( good, "Least strength required? (leave blank if it doesn't matter)")
#var powExpected %prompt( good, "Least power required? (leave blank if it doesn't matter)")
#var wisExpected %prompt( good, "Least wisdom required? (leave blank if it doesn't matter)")
#var intExpected %prompt( good, "Least intelligence required? (leave blank if it doesn't matter)")
#var agiExpected %prompt( good, "Least agility required? (leave blank if it doesn't matter)")
#var dexExpected %prompt( good, "Least dexterity required? (leave blank if it doesn't matter)")
#var conExpected %prompt( good, "Least constitution required? (leave blank if it doesn't matter)")
#var chaExpected %prompt( good, "Least charisma required? (leave blank if it doesn't matter)")
#T+ autoroller
// generate trigger
#var triggerString "#if {"
#var andRequire 0
#var numConcat 0
#var foundStatExpected 0
#if {@strExpected != ""} {
#forall @statDisplayNames {
#if {%i == @strExpected} {#var foundStatExpected 1}
#if {@foundStatExpected} {
#if {@numConcat == 0} {
triggerString = %concat( @triggerString, "(", %literal( @strength), " == "%i"")
#add numConcat 1
} {
triggerString = %concat( @triggerString, " or ", %literal( @strength), " == "%i"")
#add numConcat 1
}
}
}
#var triggerString %concat( @triggerString, ")")
#var andRequired 1
}
#var numConcat 0
#var foundStatExpected 0
#if {@powExpected != ""} {
#if {@andRequired == 1} {#var triggerString %concat( @triggerString, " and ")}
#forall @statDisplayNames {
#if {%i == @powExpected} {#var foundStatExpected 1}
#if {@foundStatExpected} {
#if {@numConcat == 0} {
#var triggerString %concat( @triggerString, "(", %literal( @power), " == ", '"', %i, '"')
#add numConcat 1
} {
#var triggerString %concat( @triggerString, "or ", %literal( @power), " == ", '"', %i, '"')
#add numConcat 1
}
}
}
#var triggerString %concat( @triggerString, ")")
#var andRequired 1
}
#var numConcat 0
#var foundStatExpected 0
#if {@wisExpected != ""} {
#if {@andRequired == 1} {#var triggerString %concat( @triggerString, " and ")}
#forall @statDisplayNames {
#if {%i == @wisExpected} {#var foundStatExpected 1}
#if {@foundStatExpected} {
#if {@numConcat == 0} {
#var triggerString %concat( @triggerString, "(", %literal( @wisdom), " == ", '"', %i, '"')
#add numConcat 1
} {
#var triggerString %concat( @triggerString, "or ", %literal( @wisdom), " == ", '"', %i, '"')
#add numConcat 1
}
}
}
#var triggerString %concat( @triggerString, ")")
#var andRequired 1
}
#var numConcat 0
#var foundStatExpected 0
#if {@intExpected != ""} {
#if {@andRequired == 1} {#var triggerString %concat( @triggerString, " and ")}
#forall @statDisplayNames {
#if {%i == @intExpected} {#var foundStatExpected 1}
#if {@foundStatExpected} {
#if {@numConcat == 0} {
#var triggerString %concat( @triggerString, "(", %literal( @intelligence), " == ", '"', %i, '"')
#add numConcat 1
} {
#var triggerString %concat( @triggerString, "or ", %literal( @intelligence), " == ", '"', %i, '"')
#add numConcat 1
}
}
}
#var triggerString %concat( @triggerString, ")")
#var andRequired 1
}
#var numConcat 0
#var foundStatExpected 0
#if {@agiExpected != ""} {
#if {@andRequired == 1} {#var triggerString %concat( @triggerString, " and ")}
#forall @statDisplayNames {
#if {%i == @agiExpected} {#var foundStatExpected 1}
#if {@foundStatExpected} {
#if {@numConcat == 0} {
#var triggerString %concat( @triggerString, "(", %literal( @agility), " == ", '"', %i, '"')
#add numConcat 1
} {
#var triggerString %concat( @triggerString, "or ", %literal( @agility), " == ", '"', %i, '"')
#add numConcat 1
}
}
}
#var triggerString %concat( @triggerString, ")")
#var andRequired 1
}
#var numConcat 0
#var foundStatExpected 0
#if {@dexExpected != ""} {
#if {@andRequired == 1} {#var triggerString %concat( @triggerString, " and ")}
#forall @statDisplayNames {
#if {%i == @dexExpected} {#var foundStatExpected 1}
#if {@foundStatExpected} {
#if {@numConcat == 0} {
#var triggerString %concat( @triggerString, "(", %literal( @dexterity), " == ", '"', %i, '"')
#add numConcat 1
} {
#var triggerString %concat( @triggerString, "or ", %literal( @dexterity), " == ", '"', %i, '"')
#add numConcat 1
}
}
}
#var triggerString %concat( @triggerString, ")")
#var andRequired 1
}
#var numConcat 0
#var foundStatExpected 0
#if {@conExpected != ""} {
#if {@andRequired == 1} {#var triggerString %concat( @triggerString, " and ")}
#forall @statDisplayNames {
#if {%i == @conExpected} {#var foundStatExpected 1}
#if {@foundStatExpected} {
#if {@numConcat == 0} {
#var triggerString %concat( @triggerString, "(", %literal( @constitution), " == ", '"', %i, '"')
#add numConcat 1
} {
#var triggerString %concat( @triggerString, "or ", %literal( @constitution), " == ", '"', %i, '"')
#add numConcat 1
}
}
}
#var triggerString %concat( @triggerString, ")")
#var andRequired 1
}
#var numConcat 0
#var foundStatExpected 0
#if {@chaExpected != ""} {
#if {@andRequired == 1} {#var triggerString %concat( @triggerString, " and ")}
#forall @statDisplayNames {
#if {%i == @chaExpected} {#var foundStatExpected 1}
#if {@foundStatExpected} {
#if {@numConcat == 0} {
#var triggerString %concat( @triggerString, "(", %literal( @charisma), " == ", '"', %i, '"')
#add numConcat 1
} {
#var triggerString %concat( @triggerString, "or ", %literal( @charisma), " == ", '"', %i, '"')
#add numConcat 1
}
}
}
#var triggerString %concat( @triggerString, ")")
#var andRequired 1
}
#var triggerString %concat( @triggerString, "} {no} {yes}")
#EXEC %concat( "#TRIGGER {^Do you want to reroll this char} {", @triggerString, "}") |
The @statDisplayNames is just a string list with all the possible names that come up. Anyways, the problem is that in the statements like triggerString = %concat( @triggerString, "(", %literal( @strength), " == "%i"") the @strength is getting expanded. I think I've narrowed it down to the = command expanding it, but I'm not sure. just showing the %concat function doesn't expand the variable, and I've also tried using the #var instead of the = command with the same results.
Putting quotes around @strength gives me almost what I want, save for the fact that it has quotes around it at the end. So I have absolutely no idea how to fix this (trolled these forums for the past 3 hours trying to find a fix for this).
Can anyone help me out on this one? |
|
|
 |
MattLofton GURU
Joined: 23 Dec 2000 Posts: 4834 Location: USA
|
Posted: Thu Apr 27, 2006 2:30 am |
you might try messing around with %expand(), but I'm not sure if that will get you what you want to be. Another thing to try is the %stripq() command that removes quotes.
|
|
_________________ EDIT: I didn't like my old signature |
|
|
 |
mabort Novice
Joined: 08 Mar 2001 Posts: 44
|
Posted: Thu Apr 27, 2006 7:23 pm |
Thanks MattLofton,
Yeah, %expand gives me crap, and %stripq ends up letting =/#var expand it afterwards.
There has to be another command that sets a variable equal to something that DOES NOT require variable expansion in the consequent.
Anyone else have any suggestions? |
|
|
 |
edb6377 Magician
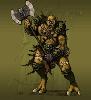
Joined: 29 Nov 2005 Posts: 482
|
Posted: Thu Apr 27, 2006 8:43 pm |
Code: |
triggerString = %concat( @triggerString, "(", %literal( @strength), " == "%i"")
|
Shouldnt that be
#var triggerString %concat( @triggerString, (", %literal( {"~@strength"), " == "%i")
or
#var triggerString %concat( @triggerString, (", %literal( {~@strength), " == "%i")
mind you this is based of a quick read i dont have time to check it right this minute. I will actually pull in the code and test it later. That would place @strength instead of the expanded value. Just a rough guess. if this doesnt work let me know and ill actually take the time to put the code into zmud and rework the entire script |
|
_________________ Confucious say "Bugs in Programs need Hammer" |
|
|
 |
|
|
|
You cannot post new topics in this forum You cannot reply to topics in this forum You cannot edit your posts in this forum You cannot delete your posts in this forum You cannot vote in polls in this forum
|
|